vue-router用法
时间: 2023-08-21 17:56:38 浏览: 106
Vue Router 是 Vue.js 官方的路由管理器,它通过路由的方式实现了前端路由的功能。它允许您在 Vue.js 应用程序中定义不同的路由,并通过这些路由来控制页面的跳转和切换。
下面是 Vue Router 的基本用法:
1. 首先,你需要安装 Vue Router。可以通过 npm 或 yarn 进行安装:
```
npm install vue-router
```
2. 在你的 Vue.js 应用程序的入口文件(通常是 main.js)中,导入 Vue Router 并使用它:
```javascript
import Vue from 'vue';
import VueRouter from 'vue-router';
Vue.use(VueRouter);
// 定义路由组件
const Home = { template: '<div>Home</div>' };
const About = { template: '<div>About</div>' };
// 定义路由
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About }
];
// 创建 router 实例
const router = new VueRouter({
routes // 在这里传入定义的路由
});
// 创建和挂载根实例
new Vue({
router
}).$mount('#app');
```
3. 在你的 Vue 组件中,使用 `<router-link>` 组件来实现路由链接,使用 `<router-view>` 组件来展示当前路由的组件:
```html
<!-- 在模板中使用路由链接 -->
<router-link to="/">Home</router-link>
<router-link to="/about">About</router-link>
<!-- 渲染当前路由匹配到的组件 -->
<router-view></router-view>
```
4. 可以在路由配置中定义更多的路由,并设置参数、嵌套路由等:
```javascript
const routes = [
{ path: '/', component: Home },
{ path: '/about', component: About },
{ path: '/user/:id', component: User },
{
path: '/nested',
component: NestedParent,
children: [
{ path: '', component: NestedDefault },
{ path: 'child', component: NestedChild }
]
}
];
```
以上是 Vue Router 的基本用法,更多高级用法,例如路由守卫、动态路由等,您可以查阅 Vue Router 的官方文档进行学习和了解。
阅读全文
相关推荐
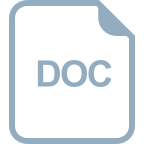
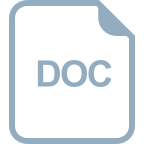
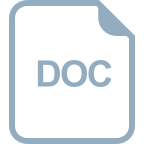
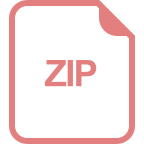
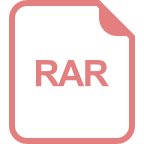

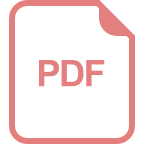
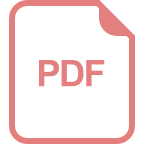
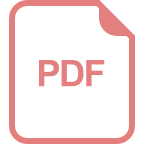
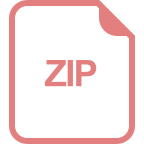
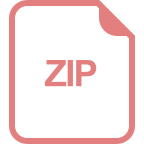
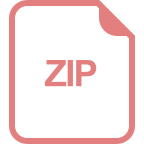
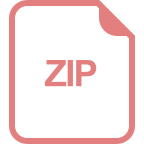
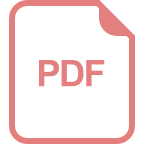
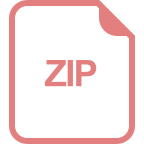
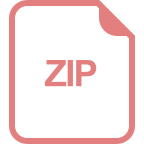


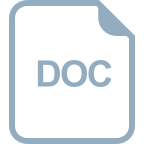