java使用Socket实现TCP协议的excel文件从服务器上传和下载到本机的开发实现
时间: 2024-10-10 11:02:07 浏览: 31
在Java中,使用Socket来实现TCP协议的Excel文件上传和下载可以分为几个步骤:
**1. 创建Server端(接收文件):**
```java
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
public class FileTransferServer {
private ServerSocket serverSocket;
public static void main(String[] args) throws IOException {
FileTransferServer server = new FileTransferServer();
server.start();
}
public void start() throws IOException {
serverSocket = new ServerSocket(8000); // 设置监听端口
System.out.println("Server started on port 8000");
while (true) {
Socket clientSocket = serverSocket.accept(); // 等待客户端连接
handleClientConnection(clientSocket);
}
}
private void handleClientConnection(Socket socket) {
try (BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true)) {
String command = in.readLine();
if ("upload".equals(command)) { // 接收命令,判断是上传还是下载
// 读取文件并发送到客户端
File fileToUpload = ...; // 获取要上传的文件
FileInputStream fis = new FileInputStream(fileToUpload);
byte[] data = new byte[(int) fileToUpload.length()];
fis.read(data);
out.println("File sent");
out.write(data);
} else if ("download".equals(command)) {
// 从本地目录读取文件,并发送给客户端
File fileToLocalDownload = ...; // 获取要下载的文件路径
FileChannel channel = new FileInputStream(fileToLocalDownload).getChannel();
out.println("Preparing to send file");
out.write(channel.map(FileChannel.MapMode.READ_ONLY, 0, fileToLocalDownload.length()));
channel.close();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
clientSocket.close();
}
}
}
```
**2. 创建Client端(上传/下载文件):**
```java
import java.io.*;
import java.net.Socket;
public class FileTransferClient {
public static void main(String[] args) {
FileTransferClient client = new FileTransferClient("localhost", 8000);
client.sendFileOrRequestFile("upload"); // 调用对应的方法进行上传
// 或者
client.sendFileOrRequestFile("download"); // 下载文件
}
private void sendFileOrRequestFile(String action) {
try (Socket socket = new Socket("localhost", 8000);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()))) {
out.println(action);
String response = in.readLine();
if ("File sent".equals(response)) { // 上传响应
// 读取响应数据并保存到本地
File file = ...; // 定义保存路径
FileOutputStream fos = new FileOutputStream(file);
fos.write(<file_data>);
fos.close();
} else if ("Preparing to send file".equals(response)) { // 下载响应
// 读取整个文件
File file = ...; // 定义临时文件保存位置
FileChannel channel = new FileOutputStream(file).getChannel();
channel.transferFrom(socket.getChannel(), 0, file.length());
channel.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,`<file_data>`应替换为实际接收到的数据。
阅读全文
相关推荐
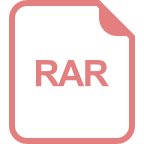
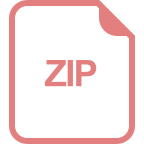
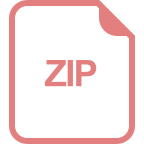















