写一个c++狼人杀小游戏代码
时间: 2024-01-08 11:21:20 浏览: 157
当然可以!下面是一个简单的C++狼人杀小游戏的代码示例:
```cpp
#include <iostream>
#include <vector>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
// 角色类
class Role {
public:
string name;
bool isWolf;
bool isDead;
Role(string n, bool w) : name(n), isWolf(w), isDead(false) {}
void kill() {
isDead = true;
}
};
// 游戏类
class WerewolfGame {
private:
vector<Role> players;
public:
void addPlayer(Role player) {
players.push_back(player);
}
void startGame() {
cout << "游戏开始!" << endl;
// 狼人杀人
for (int i = 0; i < players.size(); i++) {
if (players[i].isWolf && !players[i].isDead) {
int target = rand() % players.size(); while (players[target].isDead || players[target].isWolf) {
target = rand() % players.size();
}
players[target].kill();
cout << players[i].name << "杀死了" << players[target].name << endl;
}
}
// 显示游戏结果
cout << "游戏结束!" << endl;
for (int i = 0; i < players.size(); i++) {
cout << players[i].name << " ";
if (players[i].isDead) {
cout << "死亡";
} else {
cout << "存活";
}
cout << endl;
}
}
};
int main() {
srand(time(0));
// 创建角色
Role player1("玩家1", false);
Role player2("玩家2", false);
Role player3("玩家3", true);
Role player4("玩家4", true);
// 创建游戏
WerewolfGame game;
// 添加角色到游戏中
game.addPlayer(player1);
game.addPlayer(player2);
game.addPlayer(player3);
game.addPlayer(player4);
// 开始游戏
game.startGame();
return 0;
}
```
这个代码实现了一个简单的狼人杀小游戏。游戏中有两个狼人和两个平民,狼人会在每轮中选择一个平民进行杀死,直到所有狼人或者所有平民都死亡为止。最后会显示每个角色的生死状态。
请注意,这只是一个简单的示例代码,实际的狼人杀游戏可能需要更多的规则和功能。你可以根据自己的需求进行扩展和修改。
阅读全文
相关推荐
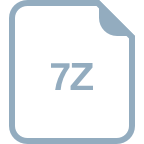
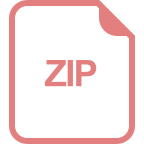
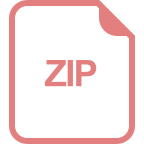
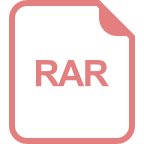
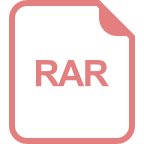
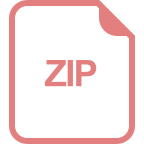
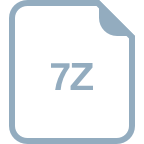
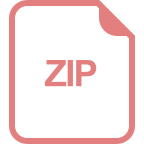
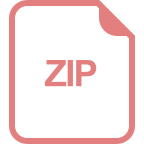
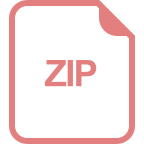
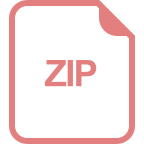
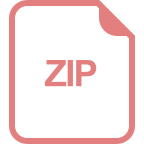
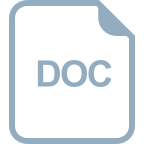