python高速下载
时间: 2024-12-31 14:32:54 浏览: 4
### 如何使用Python实现高速下载
为了提升文件下载的速度,在Python中有多种方法和技术可供选择。一种常见的做法是利用多线程或多进程来并行化下载过程,从而充分利用网络带宽。
#### 使用`requests`库与多线程组合进行分片下载
通过将大文件分割成多个部分,并发地请求这些片段可以显著加快整个下载流程。这里展示了一个简单的例子:
```python
import requests
from concurrent.futures import ThreadPoolExecutor, as_completed
def download_chunk(session, url, start_byte, end_byte, chunk_size=1024*1024):
headers = {'Range': f'bytes={start_byte}-{end_byte}'}
response = session.get(url, headers=headers, stream=True)
content = b''
for data in response.iter_content(chunk_size):
if not data:
break
content += data
return content
def multi_threaded_download(file_url, num_threads=8):
with requests.Session() as s:
head_info = s.head(file_url).headers['Content-Length']
total_length = int(head_info)
thread_pool = []
bytes_per_thread = (total_length + num_threads - 1) // num_threads
futures = []
with ThreadPoolExecutor(max_workers=num_threads) as executor:
current_pos = 0
while current_pos < total_length:
next_pos = min(current_pos + bytes_per_thread - 1, total_length - 1)
future = executor.submit(download_chunk, s, file_url, current_pos, next_pos)
futures.append(future)
current_pos = next_pos + 1
downloaded_parts = [future.result() for future in as_completed(futures)]
final_data = b''.join(downloaded_parts)
filename = "downloaded_file"
with open(filename, 'wb') as fd:
fd.write(final_data)
```
此代码段展示了如何创建一个多线程环境来进行并发HTTP GET请求以获取不同范围内的字节数据[^1]。
#### 利用专门的下载管理器如`aria2c`
对于更复杂的场景或者需要更高性能的情况,考虑采用专业的下载工具可能是更好的解决方案之一。“Aria2”是一个轻量级且功能强大的命令行下载管理器,支持HTTP/HTTPS、FTP、BitTorrent等多种协议。可以通过调用其RPC接口从Python程序控制它的工作流。
安装Aria2之后,可以在Python中配置客户端与其交互:
```python
import jsonrpcclient.http_client
# 假设 aria2 已经启动并且监听在 http://localhost:6800/jsonrpc 地址上.
client = jsonrpcclient.http_client.HttpClient('http://localhost:6800/jsonrpc')
uri = "http://example.com/largefile.zip"
result = client.request("aria2.addUri", [[uri]])
print(result.data["result"])
```
这种方法不仅能够提供更高的下载速率,还具备断点续传等功能特性[^2]。
阅读全文
相关推荐



















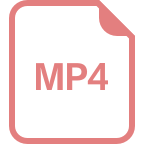