c#String 转decimal
时间: 2024-07-03 15:00:32 浏览: 156
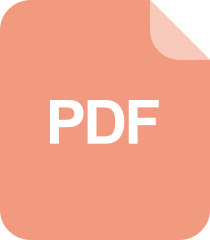
C#中decimal保留2位有效小数的实现方法
在C#中,将字符串转换为`decimal`类型需要确保字符串包含有效的数字,并且使用`Decimal.TryParse`或`Decimal.Parse`方法。下面是两种方法:
1. 使用`Decimal.TryParse`:
```csharp
string str = "123.45";
decimal decimalValue;
if (Decimal.TryParse(str, out decimalValue))
{
// 如果转换成功,decimalValue 将包含123.45
Console.WriteLine($"转换后的值: {decimalValue}");
}
else
{
// 如果转换失败,将输出错误信息,decimalValue 保持未初始化状态
Console.WriteLine("无效的字符串格式");
}
```
2. 使用`Decimal.Parse`:
```csharp
string str = "123.45";
decimalValue = Decimal.Parse(str);
// 如果字符串格式正确,decimalValue 将包含123.45
```
但是,如果字符串格式不正确(如含有非数字字符),`TryParse`会返回`false`,而`Parse`会抛出`FormatException`。
阅读全文
相关推荐
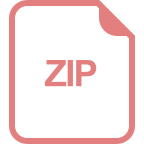
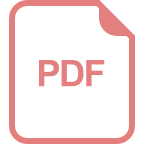















