java远程连接liux
时间: 2023-09-26 08:10:08 浏览: 52
要在Java中远程连接Linux,你可以使用SSH协议。SSH(Secure Shell)是一种加密的网络协议,用于在不安全的网络上安全地执行远程命令和文件传输。
在Java中,你可以使用JSch库来实现SSH连接。以下是一个简单的示例代码:
```java
import com.jcraft.jsch.*;
public class SSHConnection {
public static void main(String[] args) {
String host = "your_linux_host";
String username = "your_username";
String password = "your_password";
try {
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, 22);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
// 在此处执行远程命令
String command = "ls -l";
Channel channel = session.openChannel("exec");
((ChannelExec) channel).setCommand(command);
channel.setInputStream(null);
((ChannelExec) channel).setErrStream(System.err);
InputStream in = channel.getInputStream();
channel.connect();
byte[] buffer = new byte[1024];
while (true) {
while (in.available() > 0) {
int i = in.read(buffer, 0, 1024);
if (i < 0) break;
System.out.print(new String(buffer, 0, i));
}
if (channel.isClosed()) {
if (in.available() > 0) continue;
System.out.println("Exit status: " + channel.getExitStatus());
break;
}
try {
Thread.sleep(1000);
} catch (Exception ee) {
ee.printStackTrace();
}
}
channel.disconnect();
session.disconnect();
} catch (JSchException | IOException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,你需要将 `your_linux_host` 替换为你的Linux主机的IP地址或主机名,`your_username` 替换为你的用户名,`your_password` 替换为你的密码。然后,你可以在 `command` 变量中指定你要执行的远程命令。
这只是一个简单的示例,你可以根据自己的需求进行扩展和修改。另外,请确保你已经将JSch库添加到你的项目中。
请注意,远程连接Linux需要有相应的权限和配置,如允许SSH登录和正确配置防火墙等。
相关推荐
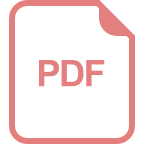
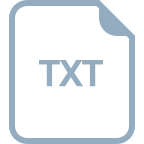
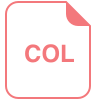
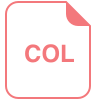
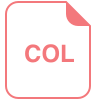
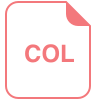
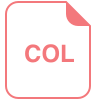









