在HTML页面中集成Leaflet库和相关的地图插件,并利用Google Maps Timeline API来加载和展示历史时期的卫星影像,创建一个交互式的地图时间线html应用。
时间: 2024-12-16 15:25:52 浏览: 12
在HTML页面中集成Leaflet地图库和Google Maps Timeline API可以按照以下步骤进行:
1. **添加必要的依赖**:
- 首先,在`<head>`部分引入Leaflet库,通常使用CDN链接:
```html
<link rel="stylesheet" href="https://unpkg.com/leaflet/dist/leaflet.css" />
<script src="https://unpkg.com/leaflet/dist/leaflet.js"></script>
```
- 同样,为了使用Google Maps Timeline API,需要引用Google Maps JavaScript API:
```html
<script async defer src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"
type="text/javascript"></script>
```
- 注:替换`YOUR_API_KEY`为你自己的Google Maps API密钥。
2. **初始化地图**:
使用Leaflet创建一个地图容器:
```html
<div id="map" style="height: 600px; width: 100%;"></div>
```
然后在JavaScript中设置地图:
```javascript
function initMap() {
var map = new L.Map('map', {
center: [51.505, -0.09], // 设置初始中心点
zoom: 8, // 初始缩放级别
});
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
}).addTo(map);
}
```
3. **加载Google Maps Timeline API**:
这个API通常用于显示基于地理位置的时间线数据。你需要获取特定时间段内的卫星影像数据,并将其作为Timeline的数据源。例如,你可以使用Google Static Maps API获取历史时期的照片。
4. **创建时间线组件**:
Google Maps Timeline API需要配合其官方提供的HTML结构和JavaScript代码来构建。创建一个时间线元素,然后在其中放置卫星图像:
```html
<div id="timeline"></div>
```
JavaScript示例:
```javascript
var timelineData = [
... // 你的卫星影像数据列表,包括时间、位置和图片URL
];
var timeline = new google.maps.Timeline(document.getElementById('timeline'), {
data: timelineData,
eventRenderers: {
marker: {
template: {
image: {
url: '{image}',
origin: new google.maps.Point(0, 0),
size: new google.maps.Size(32, 32),
scaledSize: new google.maps.Size(32, 32)
},
position: new google.maps.LatLng({lat}, {lng})
}
}
}
});
```
5. **整合两者**:
将地图容器和时间线关联起来,可能需要事件监听或数据绑定,以便当用户在时间线上选择某个时刻时,地图会自动调整到对应的位置。
```html
<!-- 添加事件监听,如用户点击时间线事件 -->
<script>
timeline.addEventListener('click', function(event) {
var latLng = event.location.latLng;
map.setView(latLng, 14); // 设置新的视图
});
</script>
```
阅读全文
相关推荐
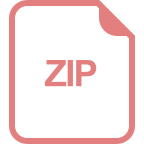
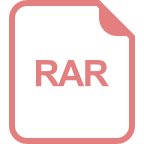
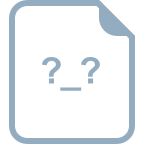















