android通过webservice连接sqlserver 详细教程
时间: 2024-01-15 21:01:44 浏览: 27
要通过Android连接SQL Server并使用WebService,您可以按照以下步骤进行操作:
1. 首先,确保您已经创建了一个用于SQL Server数据库的服务器以及相应的表。
2. 下载并安装适用于Android开发的IDE(如Android Studio)并创建新的Android项目。
3. 在Android项目的build.gradle文件中,添加Java连接SQL Server所需的依赖项。示例代码如下:
```java
dependencies {
implementation 'net.sourceforge.jtds:jtds:1.3.1'
}
```
4. 在Android的MainActivity.java文件中,编写以下代码来创建使用WebService连接SQL Server的逻辑:
```java
import android.os.AsyncTask;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class MainActivity extends AppCompatActivity {
private static final String TAG = "MainActivity";
private static final String ip = "your_server_ip_address";
private static final String port = "your_sql_server_port";
private static final String database = "your_database_name";
private static final String user = "your_username";
private static final String password = "your_password";
private Button connectButton;
private TextView resultTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
connectButton = findViewById(R.id.connectButton);
resultTextView = findViewById(R.id.resultTextView);
connectButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
new ConnectSQLServer().execute();
}
});
}
private class ConnectSQLServer extends AsyncTask<Void, Void, String> {
@Override
protected String doInBackground(Void... voids) {
String result = "";
try {
Class.forName("net.sourceforge.jtds.jdbc.Driver");
String url = "jdbc:jtds:sqlserver://" + ip + ":" + port + "/" + database;
Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("your_sql_query_here");
while (resultSet.next()) {
result += resultSet.getString("your_column_name");
}
resultSet.close();
statement.close();
connection.close();
} catch (Exception e) {
Log.e(TAG, "Error: " + e.getMessage());
result = "Error: " + e.getMessage();
}
return result;
}
@Override
protected void onPostExecute(String result) {
resultTextView.setText(result);
}
}
}
```
通过上述代码,当用户点击Connect按钮时,将会创建一个异步任务来连接SQL Server并执行查询。请确保将您的服务器IP地址、端口、数据库名称、用户名和密码替换为实际值。
5. 在Android的布局文件(activity_main.xml)中,创建一个Button和一个TextView用于显示查询结果。
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingTop="16dp"
android:paddingRight="16dp"
android:paddingBottom="16dp">
<Button
android:id="@+id/connectButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Connect"
android:layout_centerInParent="true"/>
<TextView
android:id="@+id/resultTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/connectButton"
android:textSize="18sp"/>
</RelativeLayout>
```
6. 运行Android应用程序,并点击Connect按钮以连接SQL Server并显示查询结果。
这就是使用Android连接SQL Server并使用WebService的详细教程。请注意,这只是一个基本示例,您可能需要根据您的特定需求进行一些调整。
相关推荐
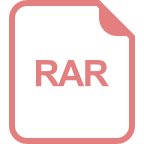
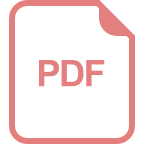














