“Hello World, this is a test.”; 查找这个字符并替换为你好 用C#正则表达式怎么写
时间: 2024-10-17 08:09:39 浏览: 18
在 C# 中,如果你想使用正则表达式查找字符串 "Hello World" 并将其替换为 "你好",你可以这样做:
```csharp
using System;
using System.Text.RegularExpressions;
public class Program {
public static void Main() {
string input = "Hello World, this is a test.";
string pattern = @"\bHello\b"; // 匹配整个单词 "Hello"
string replacement = "你好";
string result = Regex.Replace(input, pattern, replacement);
Console.WriteLine(result);
}
}
```
在这个例子中,`"\bHello\b"` 是正则表达式的模式,`\b` 表示单词边界,确保只匹配独立的 "Hello" 而不是嵌套的部分。`Regex.Replace()` 函数会找到所有匹配项并将它们替换为指定的 "你好"。
相关问题
"Hello World, this is a test."; 查找这个字符并替换为你好 用C#正则表达式怎么写
在C#中,如果你想将字符串"Hello World, this is a test."中的"Hello"替换为"你好",由于这是一个简单的单词替换,并非使用正则表达式匹配复杂的模式,可以直接使用`Substring`和`Replace`方法,不需要正则表达式。下面是示例代码:
```csharp
string inputString = "Hello World, this is a test.";
string oldWord = "Hello";
string newWord = "你好";
string outputString = inputString.Replace(oldWord, newWord);
Console.WriteLine(outputString); // 输出: 你好 World, this is a test.
```
如果你坚持要用正则表达式来做这个简单的替换,实际上并不适用,因为`"\sHello\s"`(匹配"Hello"周围有空格的情况)也不会匹配到,除非你明确指定只查找无空格的情况下"Hello"。但考虑到这里的需求并不复杂,还是建议直接使用`Replace`方法。
C#正则表达式提取括号里内容不包含括号
在C#中,你可以使用正则表达式(Regular Expression)来匹配并提取括号内的内容,但是需要注意的是,如果你想要提取的内容本身就不应该包含嵌套的括号,否则简单的正则表达式可能无法处理。下面是一个基本的例子,假设你想从字符串如 "Hello (World)" 或者 "(This is a test)" 中提取括号里的文本,而不包括内部的括号:
```csharp
using System.Text.RegularExpressions;
string input = "Hello (World)";
string pattern = @"\(([^)]+)\)";
Match match = Regex.Match(input, pattern);
if (match.Success)
{
string result = match.Groups[1].Value; // 提取第一个括号里的内容
Console.WriteLine(result); // 输出 "World"
}
else
{
Console.WriteLine("No match found.");
}
// 对于包含嵌套括号的情况,这可能需要更复杂的解析逻辑
```
这个正则表达式 `@"\(([^)]+)\)"` 匹配模式为 `(...)` 的部分,并将其中非括号字符捕获到第一个组(`\[1\]`)。
阅读全文
相关推荐
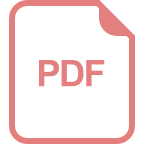
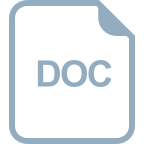
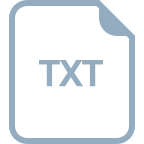
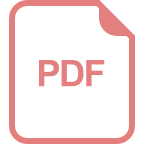
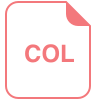
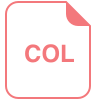
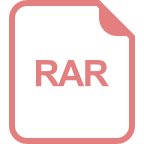
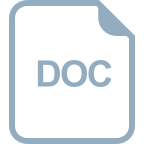
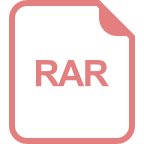
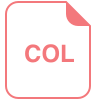




