用python代码实现:构建深度卷积神经网络。 2、利用所下载的人脸数据集和构建的深度卷积神经网络,完成人脸识别任务。
时间: 2024-12-17 07:38:22 浏览: 1
在Python中,我们可以使用深度学习框架`Keras`结合`TensorFlow`或`PyTorch`来构建深度卷积神经网络(DCNN)。以下是一个基本的例子,使用`VGGFace`预训练模型进行人脸检测和识别。请注意,这只是一个简化的示例,实际的人脸识别项目可能需要更复杂的处理,例如数据预处理、数据增强和更深层次的网络架构。
首先确保安装了必要的库:
```bash
pip install keras vggface
```
然后,你可以按照以下步骤构建和使用深度卷积网络进行人脸识别:
1. 导入所需模块:
```python
from keras.applications.vggface import VGGFace
from keras.layers import GlobalAveragePooling2D, Dropout, Flatten, Dense
from keras.models import Model
from keras.optimizers import Adam
from keras.preprocessing.image import ImageDataGenerator
from keras.utils import to_categorical
from vggface.vggface import VGGFaceModel
from PIL import Image
import os
import pickle
```
2. 加载预训练模型和人脸数据集:
```python
vgg_model = VGGFace(include_top=False, pooling='avg')
num_classes = len(os.listdir('path_to_your_dataset')) # 替换为你的数据集路径
# 加载预训练权重
vgg_model.load_weights('weights/vggface.h5')
# 将顶层替换为分类层
x = vgg_model.output
x = Flatten()(x)
x = Dense(4096, activation='relu')(x) # 添加全连接层
x = Dropout(0.5)(x)
predictions = Dense(num_classes, activation='softmax')(x)
face_recognition_model = Model(inputs=vgg_model.input, outputs=predictions)
face_recognition_model.compile(optimizer=Adam(lr=0.0001), loss='categorical_crossentropy', metrics=['accuracy'])
```
3. 数据预处理和加载:
```python
datagen = ImageDataGenerator(rescale=1./255) # 对图像缩放
train_generator = datagen.flow_from_directory('path_to_train_dataset', target_size=(224, 224), batch_size=32, class_mode='categorical')
# 加载测试数据(如果有)
test_generator = None # 根据实际情况添加
# 定义训练过程
face_recognition_model.fit(train_generator, epochs=10, validation_data=test_generator)
```
4. 测试和评估:
```python
# 使用测试数据进行预测
if test_generator is not None:
predictions = face_recognition_model.predict_generator(test_generator)
labels = test_generator.class_indices
results = [labels[np.argmax(p)] for p in predictions]
print(classification_report(test_generator.classes, results))
```
5. 保存模型:
```python
face_recognition_model.save('your_face_recognition_model.h5') # 保存模型
```
这只是一个基本的教程,实际应用可能需要更多的数据增强、迁移学习、特征提取等技术。如果你要处理更大规模的数据集,记得调整批量大小和迭代次数。
阅读全文
相关推荐
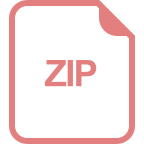
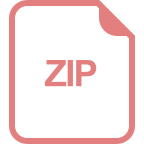
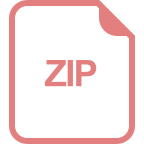















