策略模式在spring中的实现
时间: 2024-11-05 17:29:05 浏览: 7
策略模式在Spring框架中的实现通常用于创建一组相关的算法或行为,并将它们封装到独立的类中,每个类都代表一种特定的策略。在Spring中,可以使用Java配置、XML配置或者依赖注入(DI)的方式实现策略模式。
例如,你可以创建一个`PaymentStrategy`接口,包含多种支付方式:
```java
public interface PaymentStrategy {
void processPayment(double amount);
}
```
然后定义具体的策略实现,比如`CreditCardStrategy`和`PayPalStrategy`:
```java
@Component
public class CreditCardStrategy implements PaymentStrategy {
@Override
public void processPayment(double amount) {
System.out.println("Processing credit card payment for " + amount);
}
}
@Component
public class PayPalStrategy implements PaymentStrategy {
@Override
public void processPayment(double amount) {
System.out.println("Processing PayPal payment for " + amount);
}
}
```
在Spring配置文件或者Java配置类中,你可以通过`@Autowired`注解自动装配并选择合适的策略:
```xml
<bean id="paymentService" class="com.example.PaymentService">
<property name="strategy" ref="creditCardStrategy" />
</bean>
<!-- 或者在Java配置中 -->
@Autowired
private PaymentStrategy strategy; // 可以在运行时切换为PayPalStrategy
public void setStrategy(PaymentStrategy strategy) {
this.strategy = strategy;
}
public void executePayment(double amount) {
strategy.processPayment(amount);
}
```
这样,在执行`executePayment`方法时,可以根据实际需要动态地切换使用哪种策略。
阅读全文
相关推荐
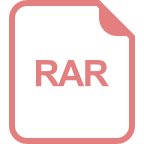
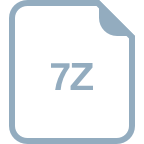
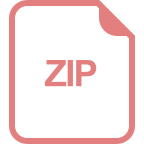















