vue的watch怎么用
时间: 2024-05-19 11:08:29 浏览: 8
在Vue中,watch是一个用于监听数据变化并执行相应操作的选项。它可以用来观察一个特定的数据属性,当该属性发生变化时,可以执行相应的回调函数。
watch选项可以在Vue组件的选项对象中定义,例如:
```javascript
export default {
data() {
return {
message: 'Hello, Vue!',
};
},
watch: {
message(newValue, oldValue) {
// 在message属性发生变化时执行回调函数
console.log('message属性发生变化');
console.log('新值:', newValue);
console.log('旧值:', oldValue);
},
},
};
```
在上面的例子中,我们定义了一个名为message的数据属性,并在watch选项中定义了一个名为message的watcher。当message属性发生变化时,watcher会执行回调函数,并传入新值和旧值作为参数。
除了基本的用法外,watch还支持更高级的用法,例如深度监听、立即执行回调函数等。你可以根据具体需求查阅Vue官方文档中关于watch的更多内容。
相关问题
vue watch 使用
Vue 的 `watch` 选项用于监听某个数据的变化,当该数据发生变化时,会执行相应的回调函数。
使用方法:
1. 在 Vue 实例的 `watch` 选项中添加需要监听的数据和对应的回调函数。
```javascript
new Vue({
data: {
message: 'Hello, Vue!'
},
watch: {
message: function (newVal, oldVal) {
console.log('message changed from', oldVal, 'to', newVal)
}
}
})
```
2. 另一种语法是使用对象形式来设置 `watch`,这种方式可以包含更多选项。
```javascript
new Vue({
data: {
message: 'Hello, Vue!'
},
watch: {
message: {
handler: function (newVal, oldVal) {
console.log('message changed from', oldVal, 'to', newVal)
},
deep: true, // 深度监听
immediate: true // 立即执行
}
}
})
```
在以上代码中,`handler` 表示回调函数,`deep` 表示是否深度监听(即深度遍历对象或数组中的所有属性),`immediate` 表示是否立即执行回调函数。
注意,`watch` 中监听的数据必须在 `data` 中声明过,否则无法监听。
当监听的数据发生变化时,回调函数会被自动调用,并传入两个参数:新值和旧值。我们可以在回调函数中执行一些逻辑操作,如更新页面显示等。
vue watch使用教程
Vue 的 watch 是一个非常常用的功能,它可以监听一个数据的变化,并在数据变化时执行相应的操作。下面是一个简单的 Vue watch 的使用教程。
假设我们有一个 Vue 组件,它有一个 data 属性叫做 message,我们想要在 message 发生变化时输出一句话。我们可以在组件的 methods 中定义一个函数来输出这句话:
```
methods: {
logMessage() {
console.log('message changed:', this.message)
}
}
```
然后,在组件的 watch 中监听 message 的变化,当 message 变化时执行 logMessage 函数:
```
watch: {
message(newValue, oldValue) {
this.logMessage()
}
}
```
这样,每当我们修改 message 的值时,都会触发 watch 中的函数,输出一句话。
除了像上面这样直接在 watch 中定义函数,我们还可以使用字符串的形式,指定一个组件中已经定义的函数来处理数据变化:
```
watch: {
message: 'logMessage'
}
```
这样,当 message 变化时,会自动调用组件中的 logMessage 函数。
除了监听单个数据的变化,我们还可以监听多个数据的变化。在 watch 中,我们可以使用对象的形式来监听多个数据的变化:
```
watch: {
message(newValue, oldValue) {
this.logMessage()
},
name(newValue, oldValue) {
this.logName()
}
}
```
这样,当 message 或者 name 发生变化时,都会触发相应的函数。
除了以上的基本用法,Vue 的 watch 还有很多高级用法,比如 deep、immediate、handler 等选项,可以满足更多的需求。需要根据具体情况进行选择使用。
相关推荐
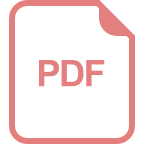
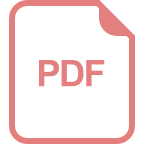
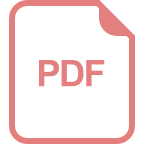
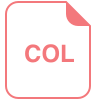
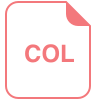
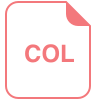
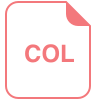
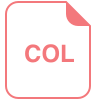








