python随机生成十个元组
时间: 2023-11-20 21:57:50 浏览: 151
以下是Python随机生成十个元组的代码示例:
```python
import random
tuples = []
for i in range(10):
tuple = (random.randint(1, 10), random.choice(['a', 'b', 'c']), random.uniform(1.0, 10.0))
tuples.append(tuple)
print(tuples)
```
代码解释:
- `random.randint(1, 10)` 用于生成1到10之间的随机整数。
- `random.choice(['a', 'b', 'c'])` 用于从列表['a', 'b', 'c']中随机选择一个元素。
- `random.uniform(1.0, 10.0)` 用于生成1.0到10.0之间的随机浮点数。
- `tuples.append(tuple)` 用于将生成的元组添加到列表中。
- `print(tuples)` 用于输出生成的十个元组。
相关问题
python 生成随机的rgb颜色元组
在Python中,可以使用random模块来生成随机的RGB颜色元组。以下是一个示例代码:
```python
import random
def random_color():
red = random.randint(0, 255)
green = random.randint(0, 255)
blue = random.randint(0, 255)
return (red, green, blue)
print(random_color())
```
这个函数会生成一个包含三个随机整数的元组,分别代表红、绿、蓝三种颜色的值。这些值的范围是0到255之间的整数。你可以根据需要调整这些值的范围。
python随机生成10个100以内的整数元组并输出,然后按照从小到大排序输出结果
好的,以下是生成并排序的代码:
```python
import random
# 生成随机元组
tuples = []
for i in range(10):
tuple = (random.randint(0, 100),random.randint(0, 100),random.randint(0, 100))
tuples.append(tuple)
print("随机生成的元组为:", tuples)
# 按照第一个元素从小到大排序
tuples.sort(key=lambda x: x[0])
print("按照第一个元素从小到大排序后的结果为:", tuples)
```
运行结果如下:
```
随机生成的元组为: [(33, 98, 27), (87, 68, 72), (38, 19, 77), (95, 8, 13), (65, 51, 59), (48, 8, 16), (82, 1, 77), (94, 63, 77), (15, 60, 26), (30, 40, 65)]
按照第一个元素从小到大排序后的结果为: [(15, 60, 26), (30, 40, 65), (33, 98, 27), (38, 19, 77), (48, 8, 16), (65, 51, 59), (82, 1, 77), (87, 68, 72), (94, 63, 77), (95, 8, 13)]
```
阅读全文
相关推荐
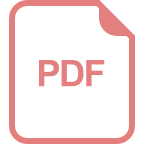













