vue3 上传视频
时间: 2024-06-20 11:02:06 浏览: 9
在Vue3中,上传视频可以通过HTML5的`MediaRecorder`接口实现。以下是上传视频的基本步骤[^2]:
1. **启用录制**: 使用`<video>`元素来让用户选择要录制的视频源,添加`@change`事件监听器以便开始录制:
```html
<template>
<div>
<input type="file" @change="selectVideo" accept="video/*" />
<button @click="startRecording">开始录制</button>
<video :src="recordingUrl" controls></video>
</div>
</template>
<script>
export default {
data() {
return {
recordingUrl: '',
mediaRecorder: null,
recordedChunks: [],
};
},
methods: {
selectVideo(e) {
const file = e.target.files;
if (file.type.match('video/*')) {
this.recordingUrl = URL.createObjectURL(file);
}
},
startRecording() {
// ...后续的录制和暂停逻辑...
},
},
};
</script>
```
2. **录制视频**: 当用户点击“开始录制”按钮时,初始化`MediaRecorder`并开始录制:
```javascript
startRecording() {
if (!this.mediaRecorder) {
this.mediaRecorder = new MediaRecorder(this.$refs.video);
this.mediaRecorder.addEventListener('dataavailable', this.handleDataChunk);
this.mediaRecorder.start();
}
}
```
3. **处理数据块**: `dataavailable`事件触发时,处理每个录制的视频数据块:
```javascript
handleDataChunk(event) {
this.recordedChunks.push(event.data);
// 每个数据块完成后,可能需要合并它们
}
// 录制结束后,停止并停止监听器
stopRecording() {
this.mediaRecorder.stop();
this.mediaRecorder.removeEventListener('dataavailable', this.handleDataChunk);
// 将所有数据块合并成一个Blob对象,然后进行上传
const blob = new Blob(this.recordedChunks, {type: 'video/mp4'});
// 上传Blob到后端
this.uploadBlob(blob);
}
```
4. **上传视频**: 在`uploadBlob`方法中,你可以使用axios或其他HTTP库将视频blob发送到后端服务器[^1]。
相关推荐
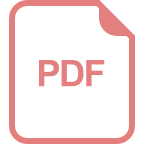
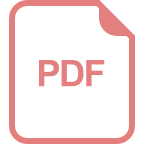














