Actually, I want to implement a resource pool, in which a counter is preserved so you can know how many places in the code where the identical resource pointer is refered. And an resource item will be recycled only when every reference to it has been released. Can you implement it in C++?
时间: 2024-04-15 19:27:46 浏览: 138
Certainly! Here is an example implementation of a resource pool in C++ that preserves a reference counter for each resource and recycles the resource only when every reference to it has been released:
```cpp
#include <iostream>
#include <unordered_map>
#include <mutex>
#include <memory>
template <typename T>
class ResourcePool {
public:
ResourcePool() {}
std::shared_ptr<T> acquire(const std::string& key) {
std::lock_guard<std::mutex> lock(mutex);
if (resources.find(key) == resources.end()) {
// Create a new resource if it doesn't exist
resources[key] = std::make_shared<Resource<T>>();
}
auto resourcePtr = resources[key];
resourcePtr->counter++;
return resourcePtr->resource;
}
void release(const std::string& key) {
std::lock_guard<std::mutex> lock(mutex);
if (resources.find(key) != resources.end()) {
auto resourcePtr = resources[key];
resourcePtr->counter--;
if (resourcePtr->counter == 0) {
// Recycle the resource if no references remain
resources.erase(key);
}
}
}
private:
template <typename U>
struct Resource {
std::shared_ptr<U> resource;
int counter;
Resource() : counter(0) {}
};
std::unordered_map<std::string, std::shared_ptr<Resource<T>>> resources;
std::mutex mutex;
};
// Example usage
class MyResource {
public:
void use() {
std::cout << "Using resource" << std::endl;
}
};
int main() {
ResourcePool<MyResource> pool;
// Acquire and use resources
for (int i = 0; i < 5; ++i) {
std::shared_ptr<MyResource> resource = pool.acquire("resource1");
resource->use();
}
// Release resources
pool.release("resource1");
return 0;
}
```
In this example, the `ResourcePool` class maintains a hash map of resource keys to shared pointers of `Resource` objects. Each `Resource` object contains the actual resource and a reference counter.
When acquiring a resource, the `acquire` function checks if the resource with the given key exists in the pool. If not, it creates a new `Resource` object and adds it to the pool. The reference counter is incremented, and the shared pointer to the resource is returned.
When releasing a resource, the `release` function decrements the reference counter of the corresponding `Resource` object. If the counter reaches zero, indicating that no more references exist, the resource is recycled by removing it from the pool.
In the example usage, we acquire and use the same resource five times using the key "resource1". After that, we release the resource using the same key. Since all references to "resource1" have been released, the resource will be recycled and removed from the pool.
Please note that this is a simplified example, and you may need to adjust it according to your specific requirements and resource management needs.
阅读全文
相关推荐
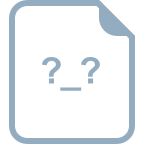

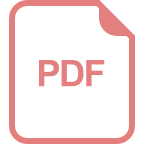







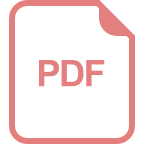





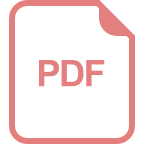
