如何在Node.js中发送HTTP请求(如GET, POST等)并获取响应?
时间: 2024-12-19 16:15:54 浏览: 3
在Node.js中,发送HTTP请求通常使用内置的`http`模块或第三方库如`axios`, `request`, 或者更现代的`node-fetch`。这里我会展示如何使用基本的`http`模块发送GET和POST请求,并接收响应。
### 发送GET请求:
```javascript
const http = require('http');
const options = {
hostname: 'example.com',
port: 80,
path: '/api/data', // API路径
};
const req = http.get(options, (res) => {
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
console.log(`GET Response: ${data}`);
});
});
req.on('error', (err) => {
console.error(`Error making GET request: ${err.message}`);
});
```
### 发送POST请求:
```javascript
const http = require('http');
const querystring = require('querystring'); // 如果需要发送JSON数据
const postData = JSON.stringify({ key: 'value' }); // 将数据转换成字符串
const options = {
hostname: 'example.com',
port: 80,
method: 'POST',
path: '/api/data',
headers: {
'Content-Type': 'application/json', // 根据需求设置正确的 Content-Type
'Content-Length': Buffer.byteLength(postData),
},
};
const req = http.request(options, (res) => {
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
console.log(`POST Response: ${data}`);
});
});
req.on('error', (err) => {
console.error(`Error making POST request: ${err.message}`);
});
// 发送POST数据
req.write(postData);
req.end();
```
**
阅读全文
相关推荐
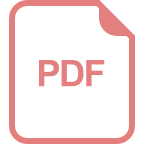
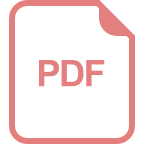
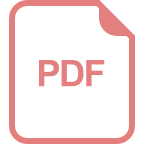















