用户输入一个英语句子《程序将字符串按分隔符(空格))进行分割,返回由单词组成的二维数组用c语言写
时间: 2024-10-14 09:01:53 浏览: 34
在C语言中,你可以使用`strtok()`函数和动态内存分配来实现这个功能。以下是一个简单的示例,展示了如何将用户输入的字符串按照空格分割成二维字符数组:
```c
#include <stdio.h>
#include <string.h>
// 助手函数,用于处理分割后的单词
char** split_string(const char* str, int* count) {
char* token = strtok(str, " "); // 使用空格作为分隔符
*count = 0;
char** arr = NULL;
while (token != NULL) {
(*count)++;
char* new_word = (char*)malloc(strlen(token) + 1); // 分配空间存储单词
strcpy(new_word, token);
if (!arr) { // 初始化数组
arr = (char**)malloc(*count * sizeof(char*));
arr[*count - 1] = new_word;
} else {
char* last = arr[(*count) - 1];
char* temp = (char*)malloc(strlen(last) + strlen(token) + 2);
strcpy(temp, last);
strcat(temp, " ");
strcat(temp, token);
free(last); // 释放旧的元素空间
arr[(*count) - 1] = temp; // 更新数组
}
token = strtok(NULL, " "); // 继续下一个单词
}
return arr;
}
int main() {
char input[1000]; // 用户输入的字符串
printf("请输入一个英文句子:");
fgets(input, sizeof(input), stdin); // 防止读取到换行符
input[strcspn(input, "\n")] = '\0'; // 去除fgets添加的换行符
int word_count;
char** words = split_string(input, &word_count);
for (int i = 0; i < word_count; i++) {
printf("单词 %d: %s\n", i+1, words[i]);
}
// 清理动态分配的内存
for (int i = 0; i < word_count; i++) {
free(words[i]);
}
free(words);
return 0;
}
阅读全文
相关推荐
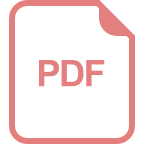
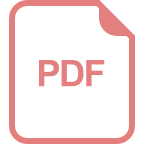
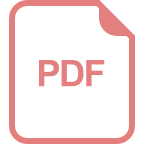





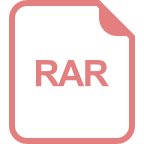









