在数据库中建立留言信息表,字段为编号、留言者、留言内容、时间。输入至少三条记录,其中包含自己的留言记录。编写JSP页面,使用JDBC技术查询显示所有留言记录。写出完整代码
时间: 2024-10-01 20:07:16 浏览: 37
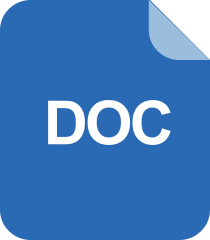
数据库课程设计之留言板

首先,我们需要创建一个名为`comments`的数据库表,包含`id`, `author`, `content`, 和 `timestamp` 字段。以下是SQL创建表的命令示例:
```sql
CREATE TABLE comments (
id INT PRIMARY KEY,
author VARCHAR(50),
content TEXT,
timestamp TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
```
然后,我们可以插入一些测试数据到表中,例如:
```sql
INSERT INTO comments (id, author, content)
VALUES (1, '张三', '这是第一条留言'),
(2, '李四', '第二条留言'),
(3, '王五', '这是我的留言');
```
接下来,我们编写JSP页面,使用JDBC查询和展示所有留言记录:
```jsp
<%@ page import="java.sql.*" %>
<html>
<body>
<table border="1">
<tr>
<th>编号</th>
<th>留言者</th>
<th>留言内容</th>
<th>时间</th>
</tr>
<%
Connection conn = null;
Statement stmt = null;
try {
// 配置数据库连接
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// 查询所有留言
stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM comments");
while (rs.next()) {
int id = rs.getInt("id");
String author = rs.getString("author");
String content = rs.getString("content");
java.util.Date timestamp = rs.getTimestamp("timestamp");
out.print("<tr>");
out.print("<td>" + id + "</td>");
out.print("<td>" + author + "</td>");
out.print("<td>" + content + "</td>");
out.print("<td>" + new java.text.SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(timestamp) + "</td>");
out.print("</tr>");
}
} catch (Exception e) {
out.println("查询错误:" + e.getMessage());
} finally {
try {
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
%>
</table>
</body>
</html>
```
注意替换`mydatabase`, `username`, 和 `password` 为你实际的数据库名、用户名和密码。
阅读全文
相关推荐
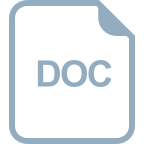
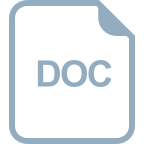
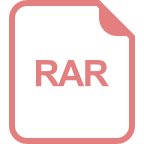
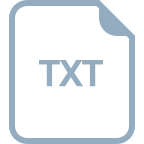
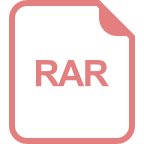
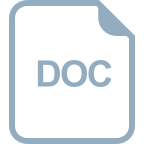
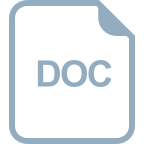
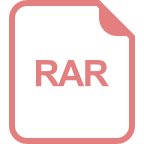
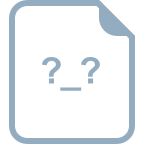
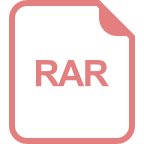
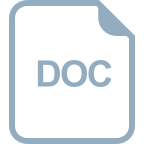
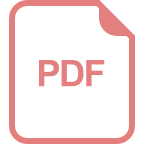
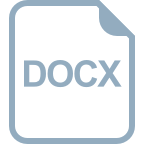
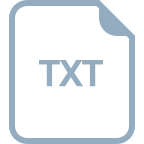