c++实现匀速贝塞尔曲线
时间: 2023-08-14 20:06:52 浏览: 140
要在C++中实现匀速贝塞尔曲线,可以使用以下步骤:
1. 定义一个结构体来表示二维点:
```cpp
struct Point {
float x;
float y;
};
```
2. 实现一个函数来计算贝塞尔曲线上的点,该函数接受起点、控制点和终点作为输入参数:
```cpp
Point calculateBezierPoint(Point p0, Point p1, Point p2, float t) {
float u = 1 - t;
float tt = t * t;
float uu = u * u;
float uuu = uu * u;
float ttt = tt * t;
Point p;
p.x = uuu * p0.x + 3 * uu * t * p1.x + 3 * u * tt * p2.x + ttt * p3.x;
p.y = uuu * p0.y + 3 * uu * t * p1.y + 3 * u * tt * p2.y + ttt * p3.y;
return p;
}
```
3. 实现一个函数来计算匀速贝塞尔曲线上的点,该函数接受起点、控制点和终点作为输入参数,并且根据给定的速度返回曲线上的点:
```cpp
Point calculateUniformBezierPoint(Point p0, Point p1, Point p2, float speed) {
float length = 0.0f;
float step = 0.001f; // 步长,可以根据需要进行调整
Point previousPoint = calculateBezierPoint(p0, p1, p2, 0);
for (float t = step; t <= 1.0f; t += step) {
Point currentPoint = calculateBezierPoint(p0, p1, p2, t);
length += sqrt((currentPoint.x - previousPoint.x) * (currentPoint.x - previousPoint.x) +
(currentPoint.y - previousPoint.y) * (currentPoint.y - previousPoint.y));
previousPoint = currentPoint;
}
float targetLength = speed * length;
float currentLength = 0.0f;
previousPoint = calculateBezierPoint(p0, p1, p2, 0);
for (float t = step; t <= 1.0f; t += step) {
Point currentPoint = calculateBezierPoint(p0, p1, p2, t);
float segmentLength = sqrt((currentPoint.x - previousPoint.x) * (currentPoint.x - previousPoint.x) +
(currentPoint.y - previousPoint.y) * (currentPoint.y - previousPoint.y));
if (currentLength + segmentLength >= targetLength) {
float ratio = (targetLength - currentLength) / segmentLength;
Point result;
result.x = previousPoint.x + (currentPoint.x - previousPoint.x) * ratio;
result.y = previousPoint.y + (currentPoint.y - previousPoint.y) * ratio;
return result;
}
currentLength += segmentLength;
previousPoint = currentPoint;
}
return calculateBezierPoint(p0, p1, p2, 1.0f);
}
```
这样,你就可以使用`calculateUniformBezierPoint`函数来计算匀速贝塞尔曲线上的点了。你需要提供起点、控制点和终点,以及期望的速度作为输入,并且函数会返回曲线上对应速度的点。
阅读全文
相关推荐




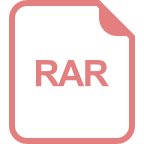









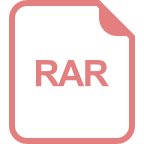