编写一个java程序,一个数组{80,92,81,89,90},去掉一个最高分和一个最低分,输出最后的平均分
时间: 2024-11-12 10:45:38 浏览: 15
在Java中,你可以按照以下步骤编写一个简单的程序来完成这个任务:
1. 首先,创建一个整数数组并初始化给定的分数。
2. 找出最高分和最低分。
3. 将这两个值从数组中移除。
4. 计算剩余分数的总和,然后除以剩下的元素个数得到平均分。
以下是示例代码:
```java
public class Main {
public static void main(String[] args) {
int[] scores = {80, 92, 81, 89, 90};
// 初始化最高分和最低分
int maxScore = scores[0], minScore = scores[0];
// 遍历数组找到最大值和最小值
for (int i = 1; i < scores.length; i++) {
if (scores[i] > maxScore) {
maxScore = scores[i];
}
if (scores[i] < minScore) {
minScore = scores[i];
}
}
// 移除最高分和最低分
for (int i = 0; i < scores.length; i++) {
if (scores[i] == maxScore || scores[i] == minScore) {
scores = removeElement(scores, i);
i--; // 更新索引,因为元素已被移除
}
}
// 计算并打印新的平均分
double averageScore = calculateAverage(scores);
System.out.println("新数组的平均分: " + averageScore);
}
// 辅助函数,从数组中删除指定位置的元素
private static int[] removeElement(int[] array, int index) {
System.arraycopy(array, index + 1, array, index, array.length - index - 1);
return Arrays.copyOfRange(array, 0, array.length - 1);
}
// 计算数组平均分
private static double calculateAverage(int[] array) {
if (array.length == 0) {
throw new IllegalArgumentException("数组为空");
}
double sum = 0;
for (int score : array) {
sum += score;
}
return sum / array.length;
}
}
```
运行此程序后,它会输出去掉了最高分和最低分后的平均分。
阅读全文
相关推荐
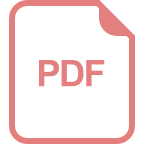
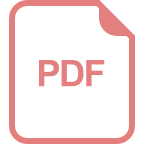
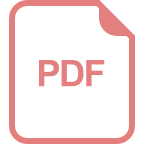















