Spring MVC项目中如何用AES256 对DB 密码加密
时间: 2024-12-17 12:36:06 浏览: 14
在Spring MVC项目中,对数据库密码进行AES256加密通常包含以下几个步骤:
1. **添加依赖**:
首先,在项目的pom.xml文件或build.gradle中添加支持AES加密的库,如BCrypt或Bouncy Castle Security Library。
```xml
<!-- Maven -->
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.68</version>
</dependency>
<!-- Gradle -->
implementation 'org.bouncycastle:bcpkix-jdk15on:1.68'
```
2. **配置环境变量**:
确保在运行环境中已经设置好Java SecureRandom所需的系统属性,例如`java.security.egd`。
3. **创建加解密工具**:
创建一个工具类,比如`PasswordEncryptor.java`,用于封装AES加密和解密操作。
```java
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import javax.crypto.Cipher;
import java.security.Key;
import java.security.Security;
public class PasswordEncryptor {
static {
Security.addProvider(new BouncyCastleProvider());
}
private static final String ALGORITHM = "AES";
private static final String KEY = "your_secret_key"; // 设置你的密钥
public static String encrypt(String password) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(password.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
public static String decrypt(String encryptedPassword) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedPassword));
return new String(decryptedBytes);
}
private static Key generateKey() throws Exception {
KeyGenerator keyGen = KeyGenerator.getInstance(ALGORITHM);
keyGen.init(256); // AES-256
SecureRandom secureRandom = SecureRandom.getInstance("SHA1PRNG");
secureRandom.setSeed(KEY.getBytes()); // 使用你的密钥生成随机数
return keyGen.generateKey();
}
}
```
4. **在DAO或Service中应用加密**:
当需要存储密码时,调用`encrypt`方法;查询时,先解密再进行比较。
```java
// DAO或Service保存密码
User user = new User();
String encryptedPassword = PasswordEncryptor.encrypt(user.getPassword());
user.setPassword(encryptedPassword);
// 查询时解密并比较
String storedPassword = ...; // 从数据库获取的加密密码
try {
if (PasswordEncryptor.decrypt(storedPassword).equals(user.getPassword())) {
// 用户名和密码匹配
}
} catch (Exception e) {
throw new RuntimeException("Failed to decrypt password", e);
}
```
阅读全文
相关推荐








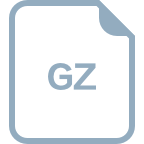


