c语言编写数据结构程序题并给出详细步骤和思路:请通过匹配找出子串T在主串S中首次出现的位置(索引从0开始),例如主串S=“abbcdefggde”,子串T=“def”,则应该返回5。
时间: 2024-11-06 20:24:09 浏览: 19
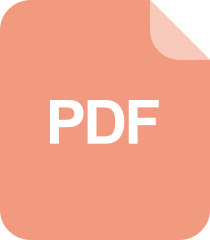
C语言数据结构之模式匹配字符串定位问题

在C语言中,你可以使用`strstr()`函数来查找子串`T`在主串`S`中首次出现的位置,这个函数会返回子串在主串中的指针,如果未找到,则返回空指针。下面是详细的步骤:
1. 定义两个字符串变量:`main_string`表示主串,`pattern`表示子串。
```c
char main_string[] = "abbcdefggde";
char pattern[] = "def";
```
2. 使用`strstr()`函数查找子串。这个函数的原型是`char *strstr(const char *haystack, const char *needle)`,它会在`haystack`中查找`needle`的第一次出现。
```c
size_t index = strstr(main_string, pattern) - main_string;
```
这里减去`main_string`是因为`strstr()`返回的是子串在主串中的起始地址,我们需要得到对应的索引。
3. 注意结果可能是`NULL`,这表示子串不存在于主串中。通常我们会对结果进行判断,如果是`NULL`,则返回-1或其他标志表示找不到。
```c
if (index == NULL)
index = -1; // 或者其他表示未找到的方式
```
综上,完整的C语言代码示例如下:
```c
#include <stdio.h>
#include <string.h>
int findSubstring(char* main_string, char* pattern) {
size_t index = strstr(main_string, pattern) -1;
return index;
}
int main() {
char main_string[] = "abbcdefggde";
char pattern[] = "def";
int result = findSubstring(main_string, pattern);
printf("The first occurrence of '%s' in '%s' is at index %d.\n", pattern, main_string, result);
return 0;
}
```
运行这段代码,你会得到输出:`The first occurrence of 'def' in 'abbcdefggde' is at index 5.`
阅读全文
相关推荐
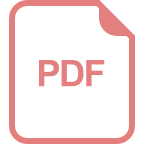
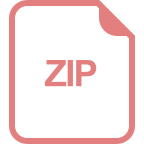
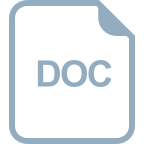
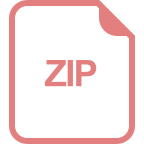
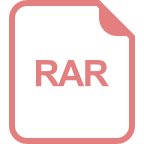
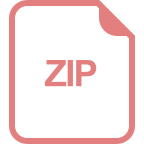
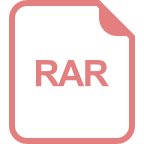
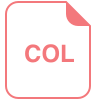
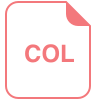
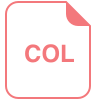
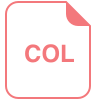
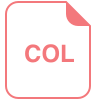
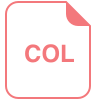
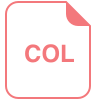
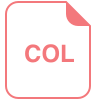
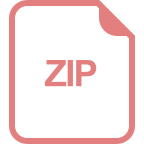
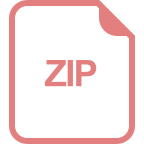
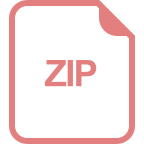