springboot整合websocket vue
时间: 2023-08-25 11:17:54 浏览: 142
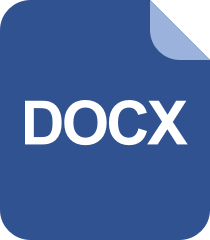
springboot整合websocket
要在Vue中使用Spring Boot整合的WebSocket,你需要使用一个WebSocket客户端库来与服务器建立连接并处理消息。在Vue中,常用的WebSocket客户端库是`vue-native-websocket`。
首先,你需要在Vue项目中安装`vue-native-websocket`依赖:
```bash
npm install vue-native-websocket
```
然后,在Vue的入口文件(通常是main.js)中引入并配置WebSocket:
```javascript
阅读全文
相关推荐
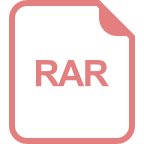
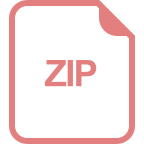
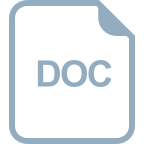


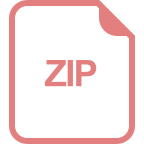
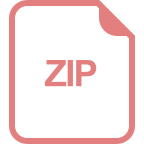
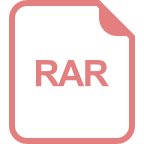
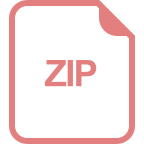
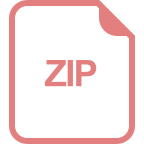
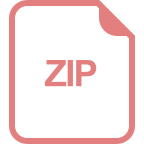
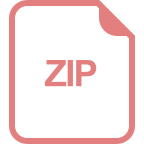
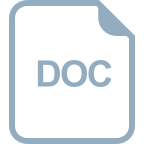
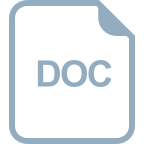
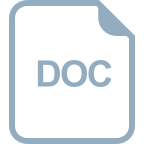
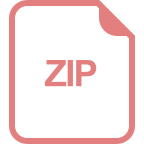
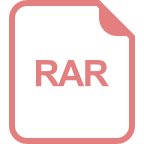
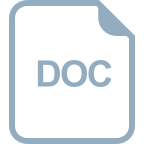