给我一个false sharing测试的C++代码
时间: 2024-09-25 16:06:37 浏览: 31
在计算机科学中,False Sharing (错误共享)是指多个线程同时访问同一块内存区域,虽然每个线程都持有这个区域的不同部分,但由于缓存一致性策略,它们可能会被分配到不同的CPU核心缓存中,这导致了看似独立的读写操作实际上会因为缓存刷新而变得相互影响,增加了竞争条件和性能开销。以下是一个简单的C++代码片段,展示如何创建一个可能导致False Sharing的情况:
```cpp
#include <thread>
#include <atomic>
#include <vector>
class SharedData {
public:
std::vector<int> data;
atomic<int> counter;
void update(int index) {
for (int i = 0; i < 100; ++i) {
data[index] += counter.fetch_add(1);
}
}
};
void thread_func(SharedData& shared_data, int threadIdx) {
for (int i = 0; i < 10; ++i) {
// 每个线程更新data的一小部分
shared_data.update(threadIdx * 10 + i);
}
}
int main() {
const int num_threads = 4;
SharedData shared;
std::vector<std::thread> threads(num_threads);
for (size_t i = 0; i < num_threads; ++i) {
threads[i] = std::thread(thread_func, std::ref(shared), i);
}
for (auto& t : threads) {
t.join();
}
return 0;
}
```
在这个例子中,`thread_func` 中的线程会并发地更新 `shared_data` 的不同元素。由于数据被分割并行处理,理论上应该减少 False Sharing,但在实际硬件上,如果缓存大小不足以存储整个数组,可能会引起这个问题。
阅读全文
相关推荐
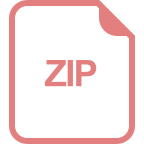
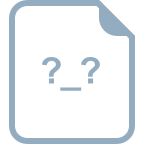
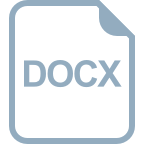
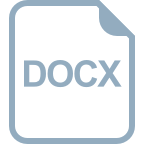
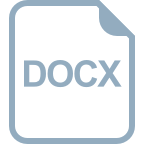
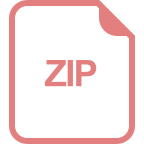
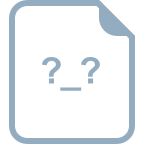