C# 找出许多点中与当前点最近的一个点
时间: 2024-12-16 07:30:18 浏览: 11
在C#中,如果你有一组二维或三维坐标点,并想要找出其中距离给定当前点最近的一个点,你可以使用空间搜索算法,如K-d树、优先队列(堆)或暴力循环计算所有点的距离并比较。这里是一个简单的示例,假设我们有Point类和List<Point>类型的点集合:
```csharp
public class Point
{
public double X { get; set; }
public double Y { get; set; } // 或者 Z for 三维
public double DistanceTo(Point other)
{
// 计算两点之间的欧氏距离
return Math.Sqrt(Math.Pow(this.X - other.X, 2) + Math.Pow(this.Y - other.Y, 2)); // 或者 Math.Pow(this.Z - other.Z, 2) for 三维
}
}
public List<Point> FindNearestPoint(List<Point> points, Point currentPoint)
{
var nearest = points.FirstOrDefault(); // 默认第一个点为最接近的
foreach (var point in points)
{
if (nearest == null || point.DistanceTo(currentPoint) < nearest.DistanceTo(currentPoint))
nearest = point;
}
return nearest;
}
```
这个函数会返回距离当前点最近的那个点。如果需要频繁查找最近点,考虑对点集构建数据结构(如K-d树)以加速查询效率。
阅读全文
相关推荐



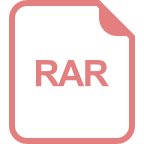



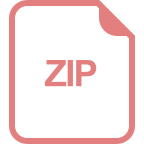


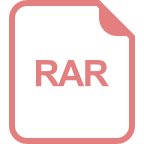
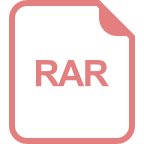

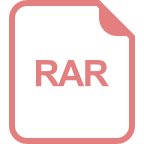

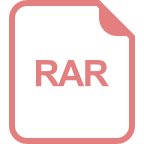