python代码实现第三章实训——动物识别系统
时间: 2024-10-21 22:14:17 浏览: 94
在Python中实现一个简单的动物识别系统,通常会涉及到图像处理和机器学习技术,特别是基于深度学习的分类模型。这里我们可以使用开源库如TensorFlow或PyTorch,并结合Keras这样的高级API来进行教学。
以下是一个基础的示例,假设我们已经有一个训练好的卷积神经网络(CNN)模型,比如VGG16或ResNet,用于识别猫狗等常见动物:
```python
# 导入所需的库
import tensorflow as tf
from tensorflow.keras.applications.vgg16 import VGG16, preprocess_input
from tensorflow.keras.preprocessing import image
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
# 加载预训练的VGG16模型,去掉顶层全连接层
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# 添加新的全局平均池化层和全连接层
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(1024, activation='relu')(x) # 输出层,假设有1000个类别,这里是简化版
predictions = Dense(num_classes, activation='softmax')(x)
# 构建完整的模型
model = Model(inputs=base_model.input, outputs=predictions)
# 冻结预训练模型的权重
for layer in model.layers[:-5]:
layer.trainable = False
# 编译模型并加载预训练权重
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 实训部分:
# 1. 遍历用户提供的图片文件,对每一幅图像进行预处理(例如归一化)
# img_path = 'path_to_image.jpg'
# img = image.load_img(img_path, target_size=(224, 224))
# x = image.img_to_array(img)
# x = np.expand_dims(x, axis=0)
# x = preprocess_input(x)
# 2. 使用模型进行预测
# preds = model.predict(x)
# predicted_class = np.argmax(preds)
# 3. 根据预测结果解释动物种类
# if predicted_class == animal_classes.index('cat'):
# print("这是一只猫")
# elif predicted_class == animal_classes.index('dog'):
# print("这是一只狗")
# ...
阅读全文
相关推荐










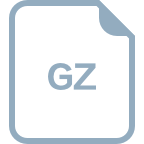







