根据以上蓝牙协议,使用uniapp写出给设备设置500米距离训练的逻辑代码
时间: 2024-12-13 20:24:11 浏览: 3
根据提供的蓝牙协议文档,我们可以编写一段uniApp代码来实现给设备设置500米距离训练的功能。以下是详细的代码示例:
```javascript
// 引入蓝牙模块
import bluetooth from '@/common/bluetooth.js';
export default {
data() {
return {
deviceId: '', // 设备ID
serviceId: '00002AD1-0000-1000-8000-00805F9B34FB', // 服务UUID
characteristicId: '00002AD9-0000-1000-8000-00805F9B34FB', // 特性UUID
distanceGoal: 500 // 设置的距离目标
};
},
methods: {
async setDistanceCourse() {
try {
// 连接设备
await this.connectDevice();
// 获取当前时间戳
const logEntryTime = Math.floor(Date.now() / 1000);
// 构建设置距离课程的payload
const payload = this.buildSetDistanceCoursePayload(logEntryTime, this.distanceGoal);
// 发送设置距离课程的指令
await this.writeCharacteristicValue(payload);
// 监听设备响应
this.listenForResponse();
} catch (error) {
console.error('设置距离课程失败:', error);
}
},
async connectDevice() {
// 打开蓝牙模块
await uni.openBluetoothAdapter();
// 开始搜索设备
await uni.startBluetoothDevicesDiscovery({ services: [this.serviceId] });
// 查找设备
const devices = await new Promise((resolve, reject) => {
uni.onBluetoothDeviceFound((res) => {
if (res.devices.length > 0) {
resolve(res.devices[0]);
} else {
reject(new Error('未找到设备'));
}
});
});
this.deviceId = devices.deviceId;
// 连接设备
await uni.createBLEConnection({ deviceId: this.deviceId });
// 获取服务
const services = await uni.getBLEDeviceServices({ deviceId: this.deviceId });
// 获取特性
const characteristics = await uni.getBLEDeviceCharacteristics({
deviceId: this.deviceId,
serviceId: this.serviceId
});
// 启用通知
await uni.notifyBLECharacteristicValueChange({
state: true,
deviceId: this.deviceId,
serviceId: this.serviceId,
characteristicId: this.characteristicId
});
},
buildSetDistanceCoursePayload(logEntryTime, distanceGoal) {
const version = 0x01; // 协议版本
const functionCode = 0x00; // 功能码 0-set
const distanceGoalLo = distanceGoal & 0xFF;
const distanceGoalHi = (distanceGoal >> 8) & 0xFF;
// 构建payload
const payload = [
0x0B, // 数据长度
0x21, // 操作码
version, // 版本
functionCode, // 功能码
...logEntryTime.toBuffer(), // 时间戳
distanceGoalLo, // 距离目标低字节
distanceGoalHi, // 距离目标高字节
0x00, 0x00 // 距离圈目标(默认0)
];
return Buffer.from(payload);
},
async writeCharacteristicValue(value) {
await uni.writeBLECharacteristicValue({
deviceId: this.deviceId,
serviceId: this.serviceId,
characteristicId: this.characteristicId,
value: value
});
},
listenForResponse() {
uni.onBLECharacteristicValueChange((res) => {
if (res.deviceId === this.deviceId && res.characteristicId === this.characteristicId) {
const response = Buffer.from(res.value);
// 解析响应
const opCodes = response[0];
const version = response[1];
const responseCode = response[response.length - 1];
if (opCodes === 0x21 && version === 0x01) {
if (responseCode === 0x00) {
console.log('设置距离课程成功');
} else if (responseCode === 0x01) {
console.error('设置距离课程失败: 错误');
} else if (responseCode === 0x02) {
console.error('设置距离课程失败: 角速度错误');
}
}
}
});
}
}
};
```
### 代码说明
1. **连接设备**:首先打开蓝牙模块,搜索设备,连接设备,并获取服务和特性。
2. **构建设置距离课程的payload**:根据协议文档中的格式构建设置距离课程的payload。
3. **发送指令**:通过`writeBLECharacteristicValue`方法将设置距离课程的指令发送到设备。
4. **监听响应**:通过`onBLECharacteristicValueChange`方法监听设备的响应,并根据响应码判断设置是否成功。
### 注意事项
- 确保设备已经正确连接并且开启了通知。
- 根据实际需求调整设备ID和服务UUID。
- 处理各种异常情况,确保代码的健壮性。
阅读全文
相关推荐
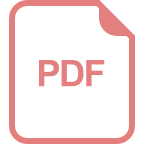
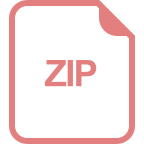
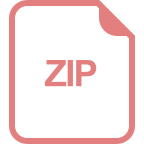

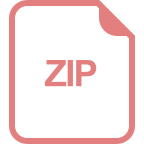
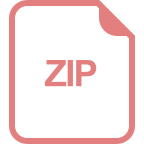
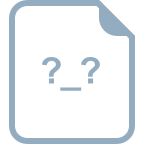
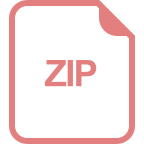
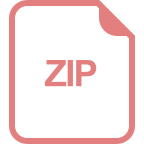
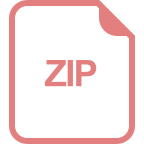



