vue外使用vuex
时间: 2023-11-08 20:54:48 浏览: 144
vuex是Vue框架中的状态管理工具,它可以帮助我们在Vue应用中共享和管理数据。在使用vuex之前,我们需要先安装vuex包,并在Vue项目中引入vuex模块。
使用vuex的步骤如下:
1. 在Vue项目中安装vuex。可以使用npm或yarn命令来安装vuex,例如:`npm install vuex`。
2. 在Vue项目的入口文件(一般是main.js)中引入vuex模块。可以使用import语句导入vuex,并通过Vue.use()方法将vuex添加为Vue的插件。例如:`import Vuex from 'vuex'` 和 `Vue.use(Vuex)`。
3. 创建一个vuex的store对象。在store对象中定义state、mutations、actions和getters等属性和方法。state用于存储数据,mutations用于修改state中的数据,actions用于处理异步操作,getters用于获取state中的数据。例如:
```
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment (state) {
state.count++
}
},
actions: {
incrementAsync ({ commit }) {
setTimeout(() => {
commit('increment')
}, 1000)
}
},
getters: {
doubleCount (state) {
return state.count * 2
}
}
})
```
4. 在Vue组件中使用vuex。可以通过this.$store来访问vuex的store对象,使用store中的state、mutations、actions和getters来处理数据。例如:
```
<template>
<div>
<p>Count: {{ $store.state.count }}</p>
<p>Double Count: {{ $store.getters.doubleCount }}</p>
<button @click="increment">Increment</button>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
export default {
methods: {
increment () {
this.$store.commit('increment')
},
incrementAsync () {
this.$store.dispatch('incrementAsync')
}
}
}
</script>
```
阅读全文
相关推荐
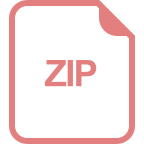
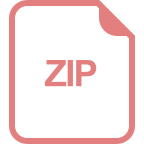
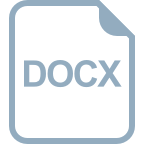
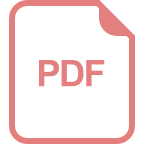
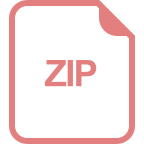
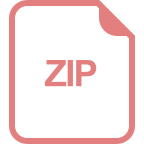
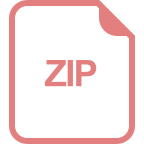
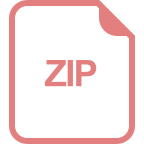
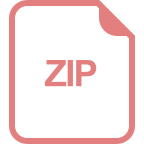
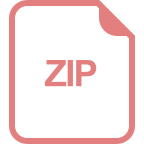
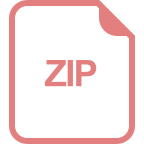
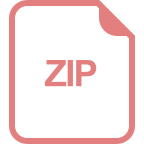
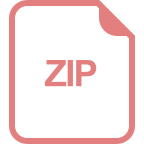
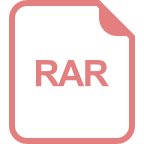
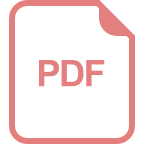

