使用Python实现对数几率回归模型 使用数据集:mnist数据集
时间: 2024-09-29 18:12:23 浏览: 51
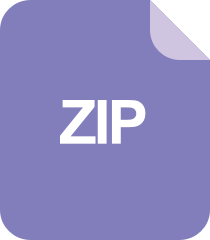
基于Python实现 MNIST 数据集分类【100011951】
在Python中,我们可以使用scikit-learn库来实现对数几率回归(Logistic Regression)模型,这是一个常用的分类算法,特别适合处理二分类问题。对于MNIST手写数字识别这样的多分类任务,虽然对数几率回归本身不是最佳选择(因为其假设特征之间独立,不适合图像这类高度相关的数据),但它仍可以用作基础模型进行学习。
首先,你需要安装必要的库,如numpy、matplotlib、pandas以及scikit-learn:
```bash
pip install numpy pandas scikit-learn matplotlib
```
接下来,你可以按照以下步骤操作:
1. **导入所需的库**:
```python
import numpy as np
import pandas as pd
from sklearn.datasets import fetch_openml
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, confusion_matrix
import matplotlib.pyplot as plt
```
2. **加载MNIST数据**:
```python
mnist = fetch_openml('mnist_784')
X, y = mnist["data"], mnist["target"]
```
3. **预处理数据**:
- 归一化数据范围
- 将标签转换为二进制表示(0或1)
```python
X = X.astype("float32") / 255.0
y = y == 0 # 对于二分类,我们只关注是否为数字0
```
4. **划分训练集和测试集**:
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
5. **创建并拟合模型**:
```python
lr = LogisticRegression()
lr.fit(X_train, y_train)
```
6. **预测和评估**:
```python
y_pred = lr.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
cm = confusion_matrix(y_test, y_pred)
print(f"Accuracy: {accuracy}")
plt.imshow(cm, cmap="Blues", interpolation="nearest")
plt.colorbar()
plt.xlabel("Predicted")
plt.ylabel("True")
plt.show()
```
阅读全文
相关推荐
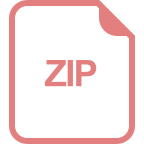
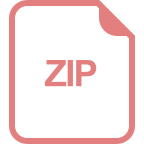
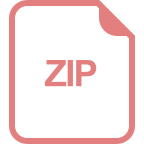
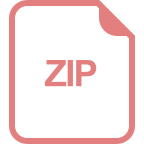
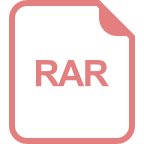
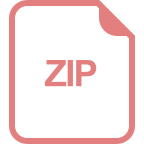
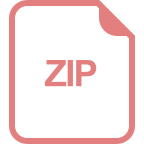
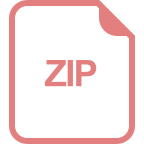
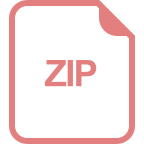
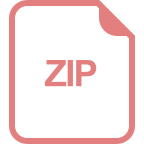
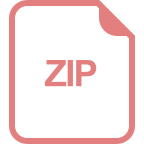
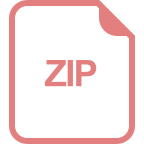
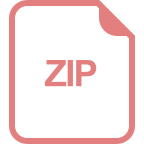
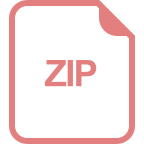
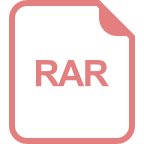
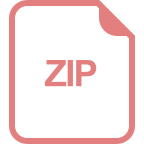
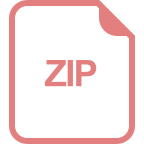
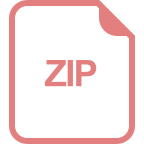