python 读取pdf内的表格
时间: 2024-09-27 22:05:01 浏览: 52
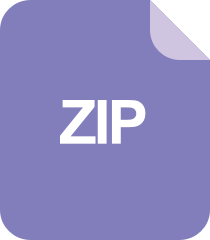
Python源码-一键提取PDF中的表格到Excel
Python读取PDF内表格通常需要借助第三方库,因为PDF并不是一种直接支持结构化数据存储的文件格式。最常用的库有`tabula-py`、`PyPDF2`配合`pandas`处理文本数据,或者使用`camelot`专门针对PDF表格提取。
以下是使用`tabula-py`和`pandas`的基本步骤:
1. 安装必要的库:
```bash
pip install tabula-py pandas
```
2. 读取PDF表格:
```python
import tabula
# 将PDF转换为DataFrame
df = tabula.read_pdf('your_pdf_file.pdf', pages='all') # 指定要读取的页数
```
如果你想要更精确地选择表格区域,可以设置参数`stream=True`和`pages`,并指定特定页面和列范围:
```python
df = tabula.read_pdf(
'your_pdf_file.pdf',
stream=True,
pages='page_number',
area=(left_column, top_row, right_column, bottom_row) # 可能需要根据实际PDF调整
)
```
3. 处理得到的DataFrame:
```python
df.head() # 查看前几行
```
阅读全文
相关推荐
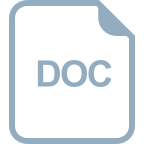
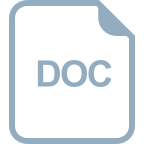
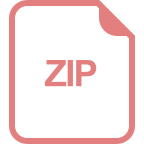














