出租车问题Python代码
时间: 2024-04-25 22:20:05 浏览: 95
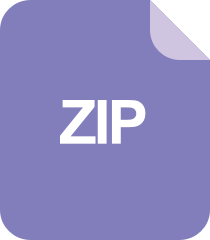
基于Python实现的武汉市出租车轨迹的数据挖掘与分析+源代码+文档说明

当然,我可以为您介绍一下出租车问题的Python代码。出租车问题是一个经典的路径规划问题,目标是找到一条最短路径从起点到终点。以下是一个简单的示例代码:
```python
import heapq
def dijkstra(graph, start, end):
# 创建一个优先队列,用于存储节点和到起点的距离
queue = [(0, start)]
# 创建一个字典,用于存储节点到起点的最短距离
distances = {start: 0}
# 创建一个字典,用于存储节点的前驱节点
predecessors = {}
while queue:
# 弹出队列中距离起点最近的节点
current_distance, current_node = heapq.heappop(queue)
# 如果当前节点已经是终点,则找到了最短路径,退出循环
if current_node == end:
break
# 遍历当前节点的邻居节点
for neighbor, weight in graph[current_node].items():
# 计算从起点到邻居节点的距离
distance = current_distance + weight
# 如果新的距离比已知的距离更短,则更新距离和前驱节点
if neighbor not in distances or distance < distances[neighbor]:
distances[neighbor] = distance
predecessors[neighbor] = current_node
# 将邻居节点和新的距离加入优先队列
heapq.heappush(queue, (distance, neighbor))
# 构建最短路径
path = []
current_node = end
while current_node != start:
path.insert(0, current_node)
current_node = predecessors[current_node]
path.insert(0, start)
return path
# 创建一个示例图
graph = {
'A': {'B': 5, 'C': 2},
'B': {'A': 5, 'D': 1, 'E': 6},
'C': {'A': 2, 'F': 8},
'D': {'B': 1, 'G': 3},
'E': {'B': 6, 'G': 2},
'F': {'C': 8, 'G': 4},
'G': {'D': 3, 'E': 2, 'F': 4}
}
start_node = 'A'
end_node = 'G'
# 调用Dijkstra算法求解最短路径
shortest_path = dijkstra(graph, start_node, end_node)
print("最短路径:", shortest_path)
```
这段代码使用了Dijkstra算法来解决出租车问题。它通过构建一个优先队列来存储节点和到起点的距离,并使用字典来存储节点到起点的最短距离和前驱节点。算法通过不断从优先队列中弹出距离起点最近的节点,并更新其邻居节点的距离和前驱节点,直到找到终点或队列为空。最后,根据前驱节点构建最短路径。
阅读全文
相关推荐
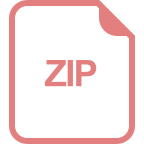
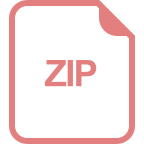
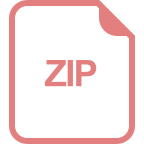













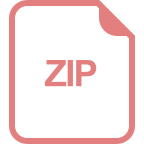
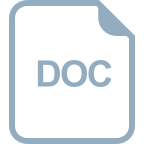