springboot 加html模版生成pdf
时间: 2024-09-20 11:15:14 浏览: 42
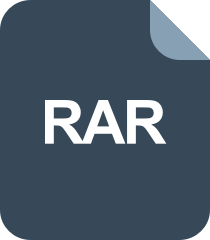
springboot+itextpdf5 通过html模板生成pdf 返回到浏览器打印
Spring Boot 和 HTML 模板结合通常用于生成 PDF 的场景,可以借助第三方库如 Thymeleaf 或 FreeMarker 来帮助实现。以下是基本步骤:
1. 添加依赖:首先,在你的 Spring Boot 项目中引入 PDF 生成库,比如 Apache Poi 或者 Flying Saucer 结合 Velocity,还有前端模板引擎相关的依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- 或者选择其他模板引擎,如 FreeMarker -->
<dependency>
<groupId>org.freemarker</groupId>
<artifactId>freemarker</artifactId>
</dependency>
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
</dependency>
```
2. 创建模板:编写 HTML 模板文件,包含你想要转换成 PDF 的内容。例如,`template.html`:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
...
</head>
<body>
<h1 th:text="${title}">Hello World</h1>
...
</body>
</html>
```
3. 编写服务:在 Spring Boot 中创建一个 Controller 或 Service 类,将 Thymeleaf 模板渲染到 PDF 上。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.thymeleaf.context.WebContext;
public class PdfController {
@Autowired
private TemplateService templateService; // 假设这个服务负责渲染和导出PDF
@GetMapping("/generate-pdf")
public ResponseEntity<byte[]> generatePdf(@RequestParam String title) {
WebContext webContext = new WebContext();
webContext.setVariable("title", title);
String templateHtml = FreeMarkerTemplateUtils.processTemplateIntoString(templateService.getTemplate(), webContext);
byte[] pdfBytes = templateService.generatePdfFromHtml(templateHtml);
return ResponseEntity.ok().contentType(MediaType.APPLICATION_OCTET_STREAM).body(pdfBytes);
}
}
```
4. 导出 PDF:最后,在 `TemplateService` 中处理模板到 PDF 的转换,这一步可能会利用 iText 或类似的库来完成。
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.pdf.PdfWriter;
public class TemplateService {
private Document document;
public String getTemplate() { ... } // 获取 HTML 模板
public byte[] generatePdfFromHtml(String htmlContent) {
try {
document = new Document();
PdfWriter.getInstance(document, new FileOutputStream("output.pdf"));
document.open();
// 将HTML内容转换为iText元素并添加到文档中
// ...
document.close();
return Files.readAllBytes(Paths.get("output.pdf")); // 返回字节流
} catch (Exception e) {
// 错误处理...
}
}
}
```
阅读全文
相关推荐
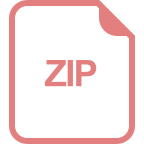
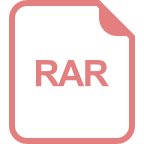

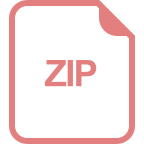
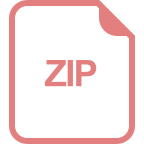
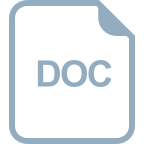
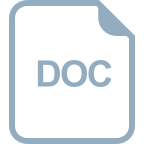





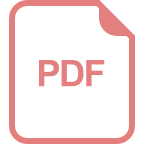
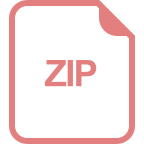
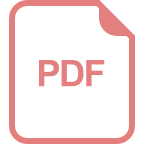

