用C语言实现统计字符串中字母、数字、空格和其他字符的个数
时间: 2024-10-26 10:16:54 浏览: 119
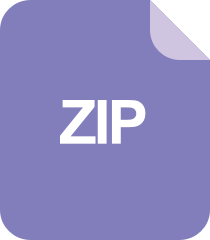
C语言统计字符串中字母、数字、空格和其他字符的个数
在C语言中,你可以使用标准库函数以及一些基本的计数技巧来统计一个字符串中各个字符类型的数量。下面是一个简单的例子,它会分别计算字母、数字、空格和其他特殊字符的数量:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void count_chars(char* str) {
int alpha_count = 0; // 字母数量
int digit_count = 0; // 数字数量
int space_count = 0; // 空格数量
int other_count = 0; // 其他字符(非字母、数字、空格)数量
while (*str != '\0') { // 遍历字符串直到遇到结束符
if (isalpha(*str)) { // 判断是否为字母
alpha_count++;
} else if (isdigit(*str)) { // 判断是否为数字
digit_count++;
} else if (*str == ' ') { // 判断是否为空格
space_count++;
} else { // 其他字符
other_count++;
}
str++; // 移动到下一个字符
}
printf("Alphabets: %d\n", alpha_count);
printf("Digits: %d\n", digit_count);
printf("Spaces: %d\n", space_count);
printf("Other characters: %d\n", other_count);
}
int main() {
char input[] = "Hello, 123 World! @example.com";
count_chars(input);
return 0;
}
```
这个程序通过`isalpha()`、`isdigit()`和`isspace()`函数来判断字符类型,并使用`while`循环遍历整个字符串。
阅读全文
相关推荐
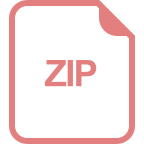
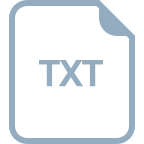















