spring boot发送邮件
时间: 2023-09-13 19:05:03 浏览: 51
你可以使用 Spring Boot 来发送邮件。首先,你需要在你的项目中添加相关的依赖。在你的 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
接下来,你需要配置邮件发送的相关信息。在 application.properties 或 application.yml 文件中添加以下配置:
```yaml
spring.mail.host=your-smtp-server
spring.mail.port=your-smtp-port
spring.mail.username=your-username
spring.mail.password=your-password
```
然后,你可以创建一个邮件服务的类来发送邮件。以下是一个简单的例子:
```java
import org.springframework.beans.factory.annotation.Autowired;import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender javaMailSender;
public void sendEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(text);
javaMailSender.send(message);
}
}
```
现在,你可以在任何地方使用 `EmailService` 类来发送邮件。例如:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@Autowired
private EmailService emailService;
@GetMapping("/send-email")
public String sendEmail() {
emailService.sendEmail("recipient@example.com", "Hello", "This is a test email.");
return "Email sent successfully.";
}
}
```
这样,当你访问 `/send-email` 路径时,就会发送一封测试邮件。记得替换上述代码中的邮件服务器和用户凭据为你自己的信息。希望这个例子对你有帮助!如有其他问题,请随时提问。
相关推荐
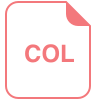
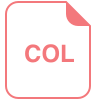
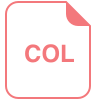
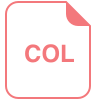
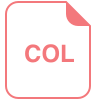









