NAR模型预测时间序列代码
时间: 2024-03-02 15:46:15 浏览: 162
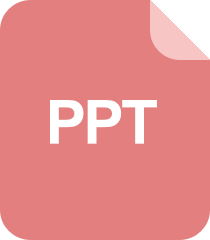
时间序列模型预测

NAR(Nonlinear AutoRegressive)模型是一种用于预测时间序列的非线性自回归模型。它基于过去的观测值来预测未来的值,并且可以捕捉到时间序列中的非线性关系。
以下是一个简单的NAR模型预测时间序列的代码示例:
```python
import numpy as np
from sklearn.neural_network import MLPRegressor
# 生成示例时间序列数据
def generate_data():
x = np.linspace(0, 10, 100)
y = np.sin(x) + np.random.normal(0, 0.1, 100)
return x, y
# 构建NAR模型
def build_nar_model(x, y):
# 将时间序列数据转换为自回归形式
lag = 5 # 自回归阶数
X = []
Y = []
for i in range(len(y) - lag):
X.append(y[i:i+lag])
Y.append(y[i+lag])
X = np.array(X)
Y = np.array(Y)
# 构建多层感知机回归模型
model = MLPRegressor(hidden_layer_sizes=(10, 10), activation='relu', solver='adam', max_iter=1000)
model.fit(X, Y)
return model
# 预测未来的时间序列值
def predict_future_values(model, x, y):
lag = 5 # 自回归阶数
last_x = y[-lag:] # 最后lag个观测值
future_x = []
for i in range(10): # 预测未来10个时间步长的值
future_y = model.predict([last_x])
future_x.append(x[-1] + (x[1] - x[0])) # 假设时间步长为常数
last_x = np.concatenate((last_x[1:], future_y), axis=0)
future_x = np.array(future_x)
return future_x
# 主函数
def main():
x, y = generate_data()
model = build_nar_model(x, y)
future_x = predict_future_values(model, x, y)
print("预测未来的时间序列值:", future_x)
if __name__ == '__main__':
main()
```
这段代码使用了Python的NumPy库和Scikit-learn库中的MLPRegressor类来构建NAR模型,并使用示例数据进行训练和预测。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
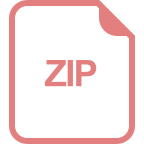
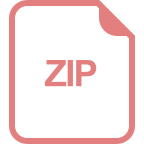


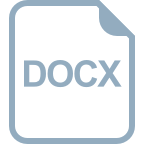
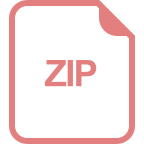
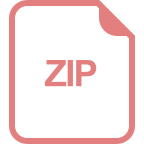
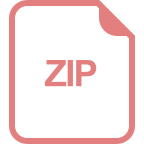
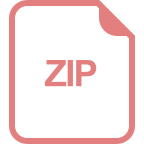
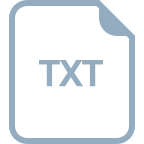
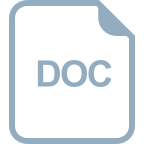
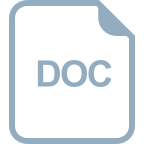
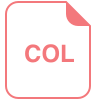
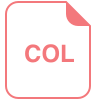
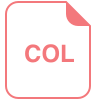

