uniapp中实现文字转语音
时间: 2024-01-28 07:04:42 浏览: 45
以下是两种在uniapp中实现文字转语音的方法:
1. 直接加语音包
```html
<!-- 在template中添加以下代码 -->
<audio id="audio"></audio>
<!-- 在script中添加以下代码 -->
export default {
methods: {
textToSpeech(text) {
const audio = document.getElementById('audio')
audio.src = `http://tts.baidu.com/text2audio?lan=zh&ie=UTF-8&text=${text}`
audio.play()
}
}
}
```
2. 采用`new SpeechSynthesisUtterance`的方式
```html
<!-- 在template中添加以下代码 -->
<button @click="textToSpeech">文字转语音</button>
<!-- 在script中添加以下代码 -->
export default {
methods: {
textToSpeech() {
const text = '需要转换为语音的文字'
const utterance = new SpeechSynthesisUtterance(text)
window.speechSynthesis.speak(utterance)
}
}
}
```
相关问题
uniapp开发app文字转语音
可以使用uniapp中的uni-speech插件来实现文字转语音功能。该插件支持多种语言和发音人选择,可以满足不同需求。
使用方法如下:
1. 在manifest.json文件中添加uni-speech插件的引用:
```
"plugins": {
"uni-speech": {
"version": "1.0.0",
"provider": "dcloud"
}
}
```
2. 在需要使用文字转语音功能的页面中,引入uni-speech插件:
```
import uniSpeech from '@/uni_modules/uni-speech/js_sdk/uni-speech.js'
```
3. 调用uniSpeech.speak方法进行文字转语音:
```
uniSpeech.speak({
text: '需要转换为语音的文字',
lang: 'zh_CN',
success: function () {
console.log('speak success');
},
fail: function (err) {
console.log('speak fail', err);
}
});
```
uniapp 文字转语音
以下是在uniapp中实现文字转语音的方法:
1.使用PDA自带的文字转语音功能,具体操作可以参考相关资料。
2.使用第三方库speak-tts,具体操作如下:
```javascript
// 安装speak-tts
npm install speak-tts
// 在需要使用的页面引入
import Speech from 'speak-tts'
// 初始化
this.speech = new Speech()
this.speech.setLanguage('zh-CN')
this.speech.init().then(() => {
console.log('语音播报初始化完成')
})
// 播放语音
this.speech.speak({text: '需要转换为语音的文字'}).then(() => {
this.speech.cancel() // 播放结束后调用
})
```
3.在微信小程序中,可以使用微信提供的插件实现文字转语音功能。
相关推荐
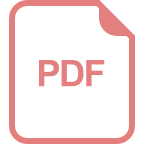
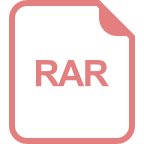












