wxpython的close怎么使用
时间: 2024-09-13 09:05:15 浏览: 25
在wxPython中,关闭一个窗口通常通过调用该窗口对象的`Close`方法来实现。当你想要关闭一个窗口时,可以调用这个方法,并且通常还会调用`Destroy`方法来确保窗口被完全销毁,释放资源。
这里有一个简单的示例来说明如何关闭一个使用wxPython创建的窗口:
```python
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
super(MyFrame, self).__init__(parent, title=title)
self.Show(True)
# 创建应用程序实例
app = wx.App(False)
# 创建窗口实例
frame = MyFrame(None, 'Sample Window')
# 运行应用程序的主事件循环
app.MainLoop()
# 关闭窗口
frame.Close()
frame.Destroy()
```
在这个例子中,`Close`方法关闭窗口,而`Destroy`方法则销毁窗口,释放系统资源。在实际应用中,你可能需要在某个事件处理器中调用这些方法,比如在用户点击窗口关闭按钮或者菜单项时。
相关问题
wxpython使用pymsql登录
首先,需要安装 PyMySQL 模块。可以通过以下命令进行安装:
```
pip install pymysql
```
然后,可以使用以下代码连接到 MySQL 数据库:
```python
import pymysql
# 连接到 MySQL 数据库
conn = pymysql.connect(
host='localhost',
user='root',
password='password',
db='database_name',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor
)
```
其中,`host`、`user`、`password` 和 `db` 分别是 MySQL 数据库的主机名、用户名、密码和数据库名称。`charset` 指定字符集为 `utf8mb4`,`cursorclass` 指定游标类型为字典类型。
接着,可以使用以下代码执行 SQL 查询并获取结果:
```python
# 创建一个游标对象
cursor = conn.cursor()
# 执行 SQL 查询
sql = "SELECT * FROM table_name"
cursor.execute(sql)
# 获取查询结果
results = cursor.fetchall()
# 关闭游标和数据库连接
cursor.close()
conn.close()
```
其中,`sql` 是要执行的 SQL 查询语句,`fetchall` 方法可以获取所有查询结果,返回一个字典列表。
最后,需要注意的是,以上代码只是连接到 MySQL 数据库和执行 SQL 查询的基本操作,在实际使用中还需要根据具体情况进行调整。
wxpython记事本
下面是一个简单的wxPython记事本的示例代码:
```python
import wx
class MyFrame(wx.Frame):
def __init__(self, parent, title):
wx.Frame.__init__(self, parent, title=title, size=(400, 400))
self.control = wx.TextCtrl(self, style=wx.TE_MULTILINE)
self.CreateStatusBar()
filemenu = wx.Menu()
menuOpen = filemenu.Append(wx.ID_OPEN, "&Open", "Open a file")
menuSave = filemenu.Append(wx.ID_SAVE, "&Save", "Save file")
menuAbout = filemenu.Append(wx.ID_ABOUT, "&About", "Information about this program")
filemenu.AppendSeparator()
menuExit = filemenu.Append(wx.ID_EXIT, "E&xit", "Terminate the program")
menuBar = wx.MenuBar()
menuBar.Append(filemenu, "&File")
self.SetMenuBar(menuBar)
self.Bind(wx.EVT_MENU, self.OnOpen, menuOpen)
self.Bind(wx.EVT_MENU, self.OnSave, menuSave)
self.Bind(wx.EVT_MENU, self.OnAbout, menuAbout)
self.Bind(wx.EVT_MENU, self.OnExit, menuExit)
self.Show(True)
def OnAbout(self,e):
dlg = wx.MessageDialog(self, " A simple text editor", "About Sample Editor", wx.OK)
dlg.ShowModal()
dlg.Destroy()
def OnExit(self,e):
self.Close(True)
def OnOpen(self,e):
""" Open a file"""
dlg = wx.FileDialog(self, "Choose a file", self.dirname, "", "*.*", wx.OPEN)
if dlg.ShowModal() == wx.ID_OK:
self.filename = dlg.GetFilename()
self.dirname = dlg.GetDirectory()
f = open(os.path.join(self.dirname, self.filename), 'r')
self.control.SetValue(f.read())
f.close()
dlg.Destroy()
def OnSave(self,e):
""" Save a file"""
dlg = wx.FileDialog(self, "Save file", self.dirname, "", "*.*", wx.SAVE | wx.OVERWRITE_PROMPT)
if dlg.ShowModal() == wx.ID_OK:
self.filename = dlg.GetFilename()
self.dirname = dlg.GetDirectory()
f = open(os.path.join(self.dirname, self.filename), 'w')
f.write(self.control.GetValue())
f.close()
dlg.Destroy()
app = wx.App(False)
frame = MyFrame(None, 'Simple Editor')
app.MainLoop()
```
这个记事本具有基本的文件打开、保存、退出和关于等功能。你可以根据需要进行修改和扩展。
相关推荐
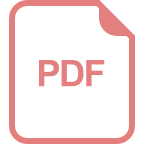
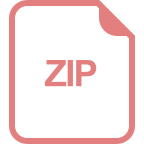
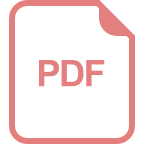












