springboot反射
时间: 2023-11-15 21:00:01 浏览: 163
SpringBoot项目采用了大量的反射机制。反射是Java语言的一种特性,它允许程序在运行时动态地获取类的信息并操作类或对象的属性、方法和构造方法等。在SpringBoot中,反射机制被广泛应用于自动配置、依赖注入等方面。通过反射,SpringBoot可以在运行时动态地加载和配置Bean,从而实现自动化配置和依赖注入。同时,SpringBoot还提供了一些工具类,如ReflectionUtils和ClassUtils等,来简化反射的使用。
相关问题
springboot反射机制
在Spring Boot中,可以使用反射机制来获取和操作Java类的信息。反射是指在程序运行时动态地获取一个类的信息,包括类名、方法名、属性名等,同时还可以调用这些方法或者修改这些属性的值。
在Spring Boot中,可以使用反射机制来实现以下功能:
1. 获取类的信息,包括类名、方法名、属性名等。
2. 动态创建对象。
3. 调用对象的方法。
4. 修改对象的属性值。
5. 获取并操作类的注解信息。
具体实现可以通过Java反射API中的Class、Method、Field、Constructor等类来实现。例如,可以使用Class.forName()方法来获取类的Class对象,然后通过Class对象的getMethod()、getDeclaredMethod()等方法来获取类的方法信息,进而调用这些方法。同时,可以使用Class的newInstance()方法来动态创建对象,或者使用Field的set()方法和get()方法来修改和获取对象的属性值。
需要注意的是,反射机制会降低程序的执行效率,因为它需要在运行时动态地获取类的信息。因此,在使用反射机制时需要注意性能问题。
springboot反射注入
在Spring Boot应用程序中使用反射注入可以方便地将依赖项注入到对象中,这可以减少手动创建依赖项实例的工作。以下是一个简单的示例:
1. 创建需要注入依赖项的类
```
public class MyService {
private MyDependency myDependency;
public void setMyDependency(MyDependency myDependency) {
this.myDependency = myDependency;
}
public void doSomething() {
myDependency.doSomethingElse();
}
}
```
2. 创建依赖项类
```
public class MyDependency {
public void doSomethingElse() {
System.out.println("Doing something else...");
}
}
```
3. 在Spring Boot应用程序中使用反射注入
```
@SpringBootApplication
public class MyApp {
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
// Get instance of MyService class using reflection
Class<?> myServiceClass = Class.forName("com.example.MyService");
Object myServiceObj = myServiceClass.newInstance();
// Get instance of MyDependency class using reflection
Class<?> myDependencyClass = Class.forName("com.example.MyDependency");
Object myDependencyObj = myDependencyClass.newInstance();
// Use reflection to call the setMyDependency method on MyService object
Method setMyDependencyMethod = myServiceClass.getMethod("setMyDependency", myDependencyClass);
setMyDependencyMethod.invoke(myServiceObj, myDependencyObj);
// Use reflection to call the doSomething method on MyService object
Method doSomethingMethod = myServiceClass.getMethod("doSomething");
doSomethingMethod.invoke(myServiceObj);
}
}
```
在这个例子中,我们使用反射获取MyService和MyDependency类的实例,然后使用反射调用setMyDependency和doSomething方法。这样,我们就可以将MyDependency实例注入到MyService实例中,并调用MyService的方法,而不需要手动创建依赖项实例。
阅读全文
相关推荐







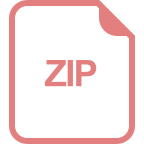
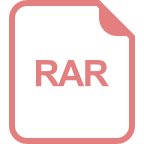
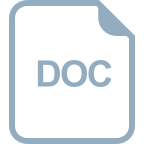




