python初学练习代码
时间: 2024-10-23 20:01:15 浏览: 16
Python 初学者可以尝试编写一些简单的代码片段来熟悉语法和基本操作。比如,你可以从打印“Hello, World!”开始,这是大多数编程语言入门的示例:
```python
print("Hello, World!")
```
接着,可以学习如何定义变量并进行数据类型转换:
```python
# 定义整数和字符串变量
num = 10
name = "Alice"
# 打印变量值
print("Number:", num)
print("Name:", name)
# 数据类型转换
age_string = str(25) # 将整数转换为字符串
print("Age as string:", age_string)
```
还可以尝试条件语句如 `if` 和 `else`:
```python
# 判断年龄是否成年
age = 18
if age >= 18:
print("You are an adult.")
else:
print("You are not yet an adult.")
```
循环结构,例如 `for` 循环遍历列表:
```python
fruits = ["apple", "banana", "orange"]
for fruit in fruits:
print(fruit)
```
函数的定义也很基础:
```python
def greet(name):
return f"Hello, {name}!"
greeting = greet("Bob")
print(greeting)
```
以上都是初级阶段的一些练习,通过这些代码,你可以逐步理解 Python 的核心概念。
阅读全文
相关推荐
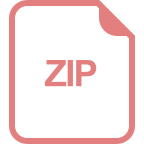
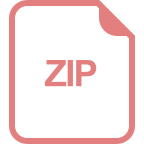
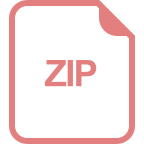
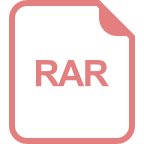
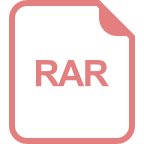
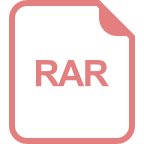
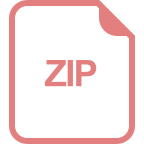
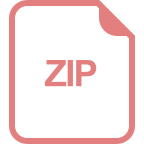
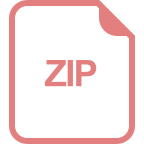
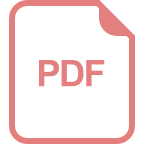
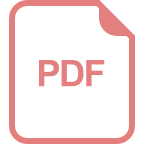
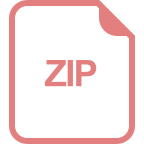
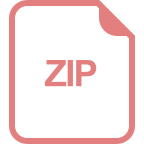
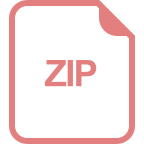
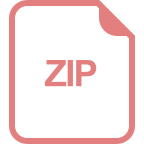
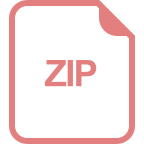
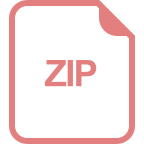
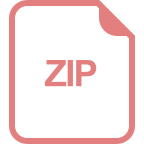