python函数的用法
时间: 2024-09-19 22:15:00 浏览: 39
在Python中,函数是一种可重复使用的代码块,它接受输入参数并返回一个值。以下是函数的基本用法:
1. **定义函数**:使用`def`关键字定义函数,后跟函数名、圆括号内的参数列表以及冒号。例如,计算两个数之和的函数可以写成:
```python
def add_numbers(a, b):
return a + b
```
2. **传递参数**:调用函数时,提供实际的参数值替换函数定义中的变量名。如 `add_numbers(3, 5)`。
3. **返回值**:函数可以有返回值,`return`语句用于结束函数并返回结果。如果没有显式返回值,则默认返回None。
4. **命名和默认参数**:你可以给参数指定默认值,如果调用时不提供,将使用默认值。比如:
```python
def greet(name="World"):
print(f"Hello, {name}!")
greet() # 输出 "Hello, World!"
greet("Alice") # 输出 "Hello, Alice!"
```
5. **可选参数和关键字参数**:还可以使用`*args`和`**kwargs`处理不定数量的位置参数和关键字参数。
6. **匿名函数**(lambda表达式):对于简单的函数,Python允许使用lambda定义,例如 `double = lambda x: x * 2`.
相关问题
vscode查看python函数用法
要在VSCode中查看Python函数的用法,可以使用以下几种方法:
1. 使用内置的IntelliSense功能:在调用函数的地方输入函数名后,按下"Ctrl" + "Space",VSCode会显示出该函数的参数和文档字符串。
2. 使用Python扩展插件:在VSCode中安装Python扩展插件后,可以使用其提供的功能来查看函数的用法。在函数名上按下"F12",即可跳转到函数的定义处;同时,可以使用"Ctrl" + "左键单击"来快速跳转到函数的定义。
3. 使用鼠标悬停:将鼠标悬停在函数名上,会显示出该函数的参数和文档字符串。
4. 使用代码导航功能:按下"Ctrl" + "Shift" + "O",在弹出的搜索框中输入函数名,可以快速定位到函数的定义处。
以上是一些常用的方法,可以帮助你在VSCode中查看Python函数的用法。
python查看函数用法
要查看Python函数的用法,可以使用内置的`help()`函数或者使用`__doc__`属性。
使用`help()`函数:
```python
help(function_name)
```
例如,要查看`range()`函数的用法,可以这样做:
```python
help(range)
```
使用`__doc__`属性:
```python
print(function_name.__doc__)
```
例如,要查看`range()`函数的用法,可以这样做:
```python
print(range.__doc__)
```
这些方法都可以帮助你查看Python函数的用法。
阅读全文
相关推荐
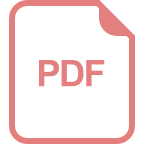
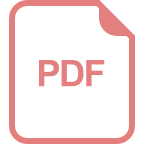
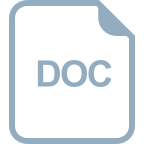
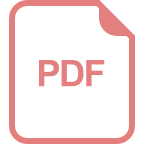
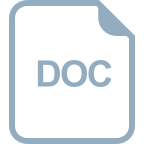
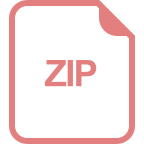
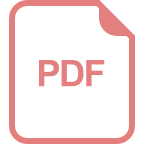
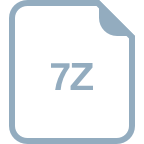
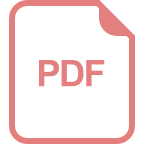
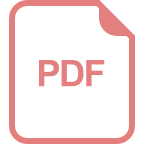
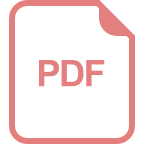
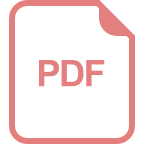
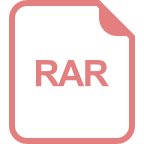
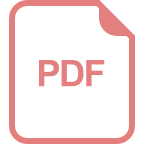
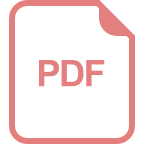