编写完整java程序计算"龟兔赛跑"的结果,龟兔赛跑的起点到终点的距离为800米,乌龟的速度为1米/1000毫秒,兔子的速度为1.2米/1000毫秒,等兔子跑到第600米时选择休息120000毫秒,请编写多线程程序计算龟兔赛跑的结果。
时间: 2024-09-18 12:19:13 浏览: 203
```java
public class TortoiseAndHareRace {
private static final int TOTAL_DISTANCE = 800;
private static final int TORTOISE_SPEED = 1 * 1000; // 1米/1000毫秒
private static final int RABBIT_SPEED = 1.2 * 1000; // 1.2米/1000毫秒
private static final int REST_TIME = 120000; // 兔子休息时间(毫秒)
public static void main(String[] args) throws InterruptedException {
Thread tortoiseThread = new Thread(() -> race(TORTOISE_SPEED, TOTAL_DISTANCE));
Thread rabbitThread = new Thread(() -> {
try {
race(RABBIT_SPEED, 600); // 兔子先跑600米
Thread.sleep(REST_TIME); // 兔子休息
race(RABBIT_SPEED, TOTAL_DISTANCE - 600); // 再跑剩余距离
} catch (InterruptedException e) {
e.printStackTrace();
}
});
tortoiseThread.start();
rabbitThread.start();
tortoiseThread.join();
rabbitThread.join();
if (tortoiseThread.isAlive() || !rabbitThread.isAlive()) {
System.out.println("比赛结果:乌龟赢得了比赛!");
} else {
System.out.println("比赛结果:兔子赢得了比赛!");
}
}
private static void race(int speed, int distance) {
long startTime = System.currentTimeMillis();
while (distance > 0) {
distance -= speed;
try {
Thread.sleep(Math.min(distance, 1000)); // 防止死循环,每次睡眠最多1秒
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.printf("乌龟进度:%d/%d, 兔子进度:%d/%d\n", distance, TOTAL_DISTANCE, distance + RABBIT_SPEED, TOTAL_DISTANCE);
}
long endTime = System.currentTimeMillis();
System.out.printf("乌龟用时:%d毫秒, 兔子用时:%d毫秒\n", endTime - startTime, System.currentTimeMillis() - startTime);
}
}
阅读全文
相关推荐
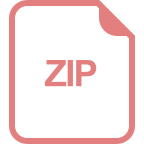
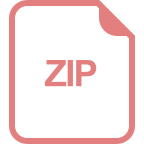
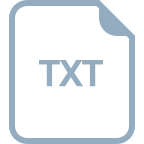















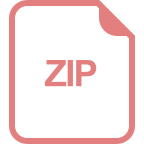
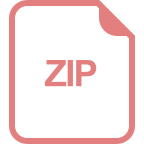