使用R语言做一个房贷计算器
时间: 2024-09-26 12:10:02 浏览: 27
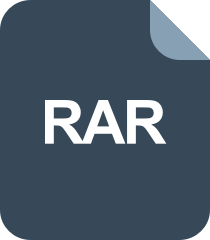
python实现房贷计算器
在R语言中制作房贷计算器,通常涉及到一些基本的数学计算和用户界面设计。以下是一个简单的步骤:
1. **安装必要的库**:
首先,你需要安装`shiny`库,它提供了一个创建交互式Web应用的基础框架。在R Studio中运行`install.packages("shiny")`安装。
2. **函数定义**:
- 创建一个函数来计算月供,输入通常是贷款金额、利率、贷款期限等。例如:
```r
mortgage_calculator <- function(principal = 1000000, rate = 0.04, term = 30) {
monthly_rate <- rate / 12
num_payments <- term * 12
payment <- principal * (monthly_rate * (1 + monthly_rate)^num_payments) / ((1 + monthly_rate)^num_payments - 1)
return(list(monthly_payment = round(payment, 2), total_paid = round(payment * num_payments, 2)))
}
```
3. **UI设计**:
使用`shiny`中的`fluidPage()`创建一个简单的网页,包括输入框和按钮。例如:
```r
ui <- fluidPage(
titlePanel("房贷计算器"),
sidebarLayout(
sidebarPanel(
numericInput("principal", "贷款金额", value = 1000000),
sliderInput("rate", "年利率", min = 0, max = 0.1, value = 0.04, step = 0.001),
numericInput("term", "贷款年限(月)", min = 12, max = 360, value = 30)
),
mainPanel(
h3("结果"),
verbatimTextOutput("output")
)
)
)
```
4. **服务器逻辑**:
通过`server()`函数处理用户的输入并显示计算结果:
```r
server <- function(input, output) {
observeEvent(input$submit_button, {
result <- mortgage_calculator(principal = input$principal,
rate = input$rate,
term = input$term)
output$output <- renderPrint(paste0("月供:$", format(result$monthly_payment, nsmall = 2),
"\n总还款额:$", format(result$total_paid, nsmall = 2)))
})
}
```
5. **运行应用**:
将`ui`和`server`合并,然后运行`shinyApp(ui, server)`。
阅读全文
相关推荐
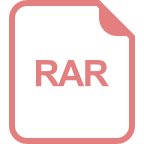
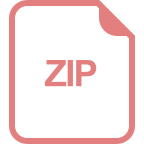
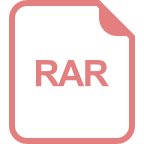
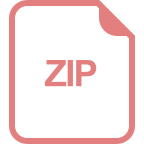
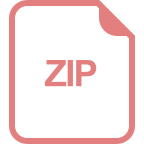
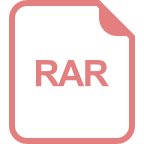
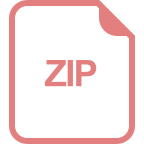
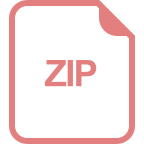
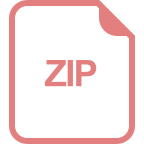
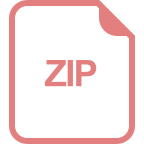
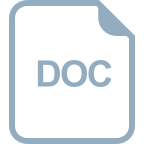
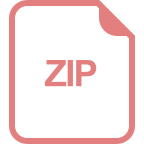
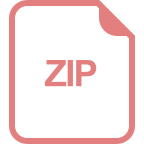