public Abstraction(Implementor implementor) { this.implementor = implementor; }
时间: 2024-12-18 16:33:54 浏览: 5
这段代码是Java中创建一个抽象类(Abstraction)的构造函数,其中`implementor`是一个实现了`Implementor`接口的对象。通过这种方式,抽象类`Abstraction`可以依赖于具体的实现类(`Implementor`),这体现了设计模式中的"依赖倒置原则"(Dependency Inversion Principle,DIP)。当需要改变`Implementor`的行为时,只需要更换实现类即可,而不需要修改`Abstraction`本身,提高了代码的灵活性。
这个构造函数的大致作用是,在创建`Abstraction`实例的时候,指定它所关联的具体实现。例如:
```java
public class ConcreteImplementor implements Implementor {
// 实现体...
}
// 使用构造函数
AbstractAbstraction abstraction = new Abstraction(new ConcreteImplementor());
```
在这个例子中,`abstraction`将使用`ConcreteImplementor`的实现来完成其功能。
相关问题
Implement time_per_word, which takes in times_per_player, a list of lists for each player with timestamps indicating when each player finished typing each word. It also takes in a list words. It returns a game with the given information. A game is a data abstraction that has a list of words and times. The times are stored as a list of lists of how long it took each player to type each word. times[i][j] indicates how long it took player i to type word j. For example, if times_per_player = [[1, 3, 5], [2, 5, 6]], the corresponding time attribute of the game would be [[2, 2], [3, 1]]. Timestamps are cumulative and always increasing, while the values in time are differences between consecutive timestamps. Be sure to use the game constructor when returning a game, rather than assuming a particular data format.Given timing data, return a game data abstraction, which contains a list of words and the amount of time each player took to type each word. Arguments: times_per_player: A list of lists of timestamps including the time the player started typing, followed by the time the player finished typing each word. words: a list of words, in the order they are typed.
Here's a possible implementation of the `time_per_word` function:
```python
from typing import List
from dataclasses import dataclass
@dataclass
class Game:
words: List[str]
times: List[List[int]]
def time_per_word(times_per_player: List[List[int]], words: List[str]) -> Game:
n_players = len(times_per_player)
n_words = len(words)
times = [[0] * (n_words - 1) for _ in range(n_players)]
for i in range(n_players):
for j in range(1, n_words):
times[i][j - 1] = times_per_player[i][j] - times_per_player[i][j - 1]
return Game(words=words, times=times)
```
The function takes in `times_per_player`, a list of lists where each inner list represents the timestamps for a single player, and `words`, a list of strings representing the words in the order they were typed. It returns a `Game` object containing the words and the time each player took to type each word.
The implementation uses a nested loop to iterate over all pairs of players and words, and computes the time difference between consecutive timestamps for each player and word. The resulting `times` matrix has dimensions `(n_players, n_words - 1)` to account for the fact that the first timestamp only indicates when typing started, not when the first word was completed. The `Game` object is constructed using the `dataclass` decorator from Python 3.7+ to create a simple data structure with named fields.
Note that this implementation assumes that all players typed the same set of words in the same order, and that the timestamps are cumulative and increasing (i.e., the time to type each word is always positive). If these assumptions don't hold, the function may produce incorrect results or raise errors.
I'd like to implement a DMA assistant software that can implement the perspective function of the game, and what is the solution
Implementing a DMA (Direct Memory Access) assistant software to handle perspective transformations for a game would require leveraging hardware acceleration where available and optimizing the data transfer process. Here's a simplified approach using Python-like pseudocode for explanation, keeping in mind actual implementation might involve C/C++ or low-level APIs for games:
1. **Using an Existing API**: Many gaming engines such as OpenGL or Vulkan already have built-in functions like `glRotate`, `glTranslate`, `or glOrtho`[^4], to perform 2D or 3D perspective transformation. For simple cases, these can be accessed directly by setting up vertex buffers and enabling vertex transformation.
```python
# For an engine with proper abstraction like PyOpenGL
from OpenGL.GL import *
# Create your vertices array with transformed positions
transformed_vertices = perspective_transform(original_vertices)
# Bind and update the VBO (Vertex Buffer Object)
vertex_buffer.data = transformed_vertices
glEnableClientState(GL_VERTEX_ARRAY)
glVertexPointer(*vertex_format)
```
2. **DMA for Data Transfer**: If you need to optimize data streaming during real-time updates, you may use hardware-based Direct Memory Copy[^5]. This will allow the graphics card itself to copy data between memory without involving CPU heavily, hence improving performance.
```markdown
```c
glCopyBufferSubData(source_texture, destination_texture, source_offset, destination_offset, bytes_to_transfer);
```
3. **Multi-threading**: For handling complex rendering pipelines where multiple perspectives are involved, consider offloading tasks to separate threads, which can improve overall system efficiency[^6].
4. **Hardware Acceleration:** Make use of dedicated GPU's parallel processing capabilities through Compute Shaders[^7] if you need high-performance parallel rendering.
```python
# (C++) Example of compute shader performing transformations
GLuint shader Program;
...
glUseProgram(program);
glBindImageTexture(unit位置, textureHandle, level, GL_TRUE, 0 /* mipmap level */, format, accessMode);
// Call the kernel to perform perspective transform on a buffer within shader
kernel<<<gridSize.width, gridSize.height>>>(bufferWidth, bufferHeight);
GLint status;
glGetUniformLocation(shader Program, "u_perspective_matrix");
glUniformMatrix4fv(positionsBufferLocation, 1, GL_FALSE, &perspectiveMatrix);
```
阅读全文
相关推荐
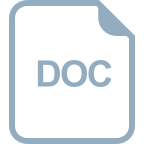
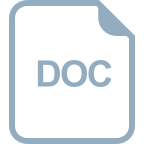
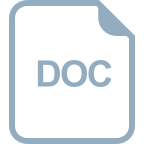
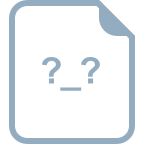
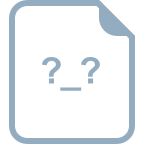
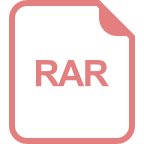
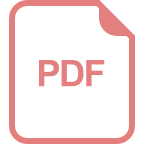
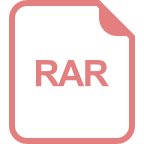
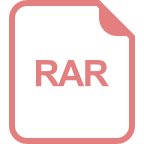
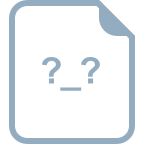
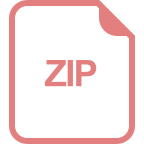
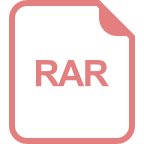
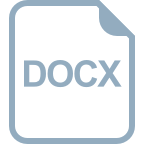
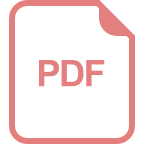
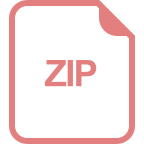
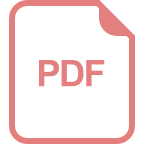
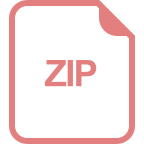