使用scapy实现icmp扫描
时间: 2024-10-19 13:06:15 浏览: 52
在Scapy中,你可以使用`ICMP`模块来实现ICMP(Internet Control Message Protocol)扫描,特别是ping扫描,即检查目标主机是否在线。这里有一个基本的示例,展示如何发送ICMP echo request (ping) 并接收回应:
```python
from scapy.all import ICMP, srp
def icmp_scan(ip_address):
packets = ICMP(type="echo-request", id=42, data="Hello, World!") / IP(dst=ip_address)
responses, _ = srp(packets, timeout=2, verbose=0)
for sent, received in responses:
if received.type == "echo-reply":
print(f"{ip_address} is up and responded to ping.")
else:
print(f"{ip_address} did not respond or unreachable.")
# 使用你的目标IP替换这里的字符串
icmp_scan("192.168.1.1")
相关问题
利用scapy实现IP存活扫描器
Scapy是一个强大的网络协议分析工具,它在Python中提供了一个接口,可以用于创建、发送和解析各种网络数据包,包括IP存活扫描。IP存活扫描的基本原理是向目标主机发送ICMP(Internet Control Message Protocol)的ping请求,如果收到回应,就表明目标主机在线。
在Scapy中实现IP存活扫描器的一般步骤如下:
1. 导入必要的模块:
```python
from scapy.all import IP, ICMP, sr
```
2. 定义要扫描的目标列表:
```python
targets = ["192.168.1.1", "192.168.1.2", ...]
```
3. 创建一个封装了ICMP ping请求的数据包:
```python
def create_ping_packet(destination):
packet = IP(dst=destination) / ICMP()
return packet
```
4. 发送ping请求并处理响应:
```python
def perform_scan(targets):
for target in targets:
response, _ = sr(create_ping_packet(target), timeout=2, verbose=False)
if response:
print(f"{target} is alive")
else:
print(f"{target} seems to be offline or not responding")
```
5. 调用扫描函数:
```python
perform_scan(targets)
```
使用scapy包以及icmp协议,实现一个局域网全IP地址扫描器,扫描出本网段所有在线主机,并打印IP地址到屏幕上。
以下是使用scapy包和icmp协议实现局域网全IP地址扫描器的Python代码:
```python
from scapy.all import *
def scan_network(ip):
# 构造icmp请求包
packet = IP(dst=ip)/ICMP()
# 发送icmp请求包并等待响应
response = sr1(packet, timeout=1, verbose=0)
# 如果有响应,则表示该主机在线
if response:
print(ip)
# 获取本机IP地址和子网掩码
conf.verb = 0
ip, subnet_mask = sr1(IP(dst="google.com")/ICMP(), verbose=0).src, get_if_raw_hwaddr(conf.iface)[1]
# 计算本网段IP地址范围
network_address = str(IPAddress(ip) & IPAddress(subnet_mask))
broadcast_address = str(IPAddress(ip) | IPAddress(~subnet_mask))
ip_range = [str(x) for x in range(int(network_address)+1, int(broadcast_address)-1)]
# 扫描本网段所有IP地址
for ip in ip_range:
scan_network(ip)
```
首先,我们需要使用scapy包中的sr1函数来发送icmp请求包并等待响应。如果有响应,则表示该主机在线,我们就可以将其IP地址打印到屏幕上。
为了扫描本网段所有IP地址,我们需要获取本机IP地址和子网掩码,并计算出本网段IP地址范围。这可以通过发送ICMP请求包到任意外部IP地址来获取本机IP地址和子网掩码,然后使用IPAddress类计算出本网段IP地址范围。
最后,我们循环遍历IP地址范围,并调用scan_network函数来扫描每个IP地址。注意,我们需要将conf.verb设置为0来禁止scapy输出详细的调试信息,以减少不必要的干扰。
阅读全文
相关推荐
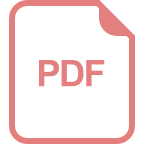
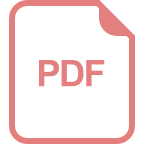
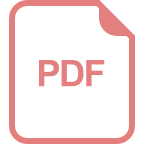
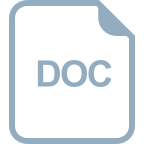




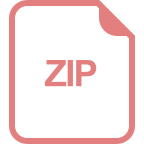
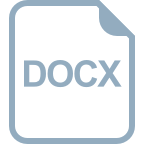
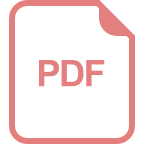
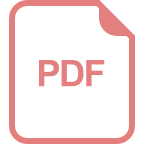
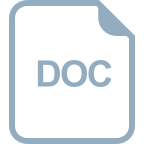
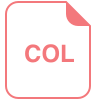


