数据结构:删除非递减的线性表中相同的元素。写出代码
时间: 2024-10-28 22:07:42 浏览: 12
在非递减的线性表(即有序表)中删除相同的元素,可以采用迭代的方式来实现,避免直接修改原列表导致的复杂性。这里提供一个简单的 Python 示例,使用两个指针,一个遍历表,另一个记录当前的唯一值:
```python
def remove_duplicates(head):
if not head or not head.next:
return head
current = head
while current and current.next:
if current.value == current.next.value:
current.next = current.next.next
else:
current = current.next
return head
```
在这个函数中,`head` 是线性表的头结点,`current` 指针用于遍历,如果遇到两个连续的节点值相等,则将第二个节点跳过;否则,`current` 向前移动到下一个节点。
注意:这里的 `value` 是假设线性表节点有一个名为 `value` 的属性,表示节点的值。实际的实现可能会根据你的数据结构和语言略有差异。
相关问题
线性表中数据元素的值为正整数,若线性表采用带头结点的单链表结构,请创建一个非递减有序的单链表,请用c语言写出这个算法
在C语言中,创建一个非递减有序的单链表需要遍历链表并插入新节点以保持顺序。这里提供一个基本的算法步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个新的链表节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 插入节点以保持非递减顺序
void insertSorted(Node** head, int value) {
Node* current = *head;
if (*head == NULL || value <= current->data) {
// 如果链表为空或新值小于等于当前节点,直接插入
newNode->next = *head;
*head = newNode;
return;
}
while (current->next != NULL && current->next->data < value) {
current = current->next; // 遍历到适当位置
}
newNode->next = current->next; // 插入新节点
current->next = newNode;
}
// 打印链表
void printList(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL; // 初始化链表头指针
// 添加一些节点,例如从最小到最大
for (int i = 1; i <= 10; ++i) {
insertSorted(&head, i);
}
// 打印链表确认是否排序
printList(head);
return 0;
}
```
注意,这只是一个基础示例,实际应用中可能需要考虑更多边界情况和错误处理。运行这个程序会创建一个非递减有序的链表,其中包含正整数。
Python已知线性表la 和 lb 中的数据元素按值非递减有序排列,现要求将La和lb归并为一个新的线性表lc,且lc中的数据元素仍按值非递减有序排列。且要求不能有重复元素。 请写出对应代码,
在Python中,你可以使用内置的`heapq`库或者简单的迭代合并策略来实现这个任务。这里我们提供一种基于堆排序的解决方案,因为它能够保证结果的非递减有序且没有重复元素。
```python
import heapq
def merge_sorted_lists(la, lb):
# 创建两个空堆,大顶堆存储la,小顶堆存储lb,保证不会重复
h1, h2 = [], []
for item in la:
heapq.heappush(h1, -item)
for item in lb:
heapq.heappush(h2, item)
# 初始化结果列表
lc = []
while h1 and h2:
if -h1[0] <= h2[0]: # 如果la的大顶堆元素不大于lb的小顶堆元素
lc.append(-heapq.heappop(h1)) # 取la的大顶堆元素并添加到lc,记得翻转负号
else:
lc.append(heapq.heappop(h2))
# 将剩余元素添加到lc
lc.extend(h1) if h1 else lc.extend(h2)
return lc
# 示例
la = [1, 3, 5]
lb = [2, 4, 6]
lc = merge_sorted_lists(la, lb)
print(lc) # 输出:[1, 2, 3, 4, 5, 6]
```
这个函数首先将输入的两个有序列表转换成堆结构,然后通过不断地比较堆顶元素并取较小的一个来合并。最后,如果其中一个堆为空,就直接将另一个堆的内容添加到结果列表中。
阅读全文
相关推荐
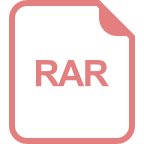
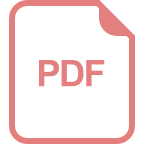
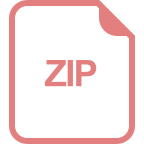
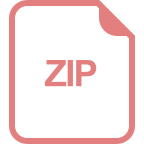
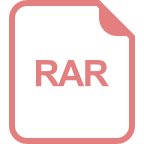
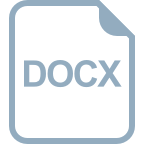
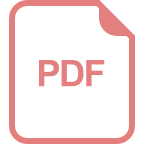
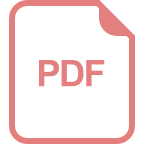
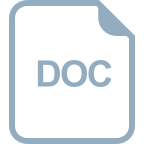
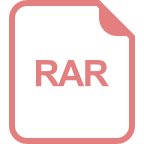
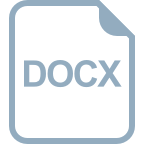
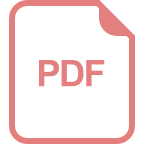
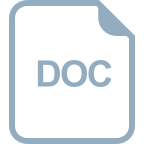
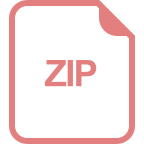
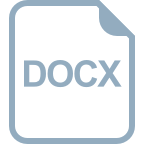