用c语言由键盘输入一个华氏温度,求摄氏温度。输出要有说明,取两位小数。转换公式为:摄氏温度=5/9*例:运行时从键盘输入46 运行结果为:The Celsius temperature is 7.78
时间: 2024-09-13 07:09:20 浏览: 46
在C语言中,你可以通过以下步骤实现这个功能:
```c
#include <stdio.h>
// 函数声明,用于将华氏温度转换为摄氏温度
double fahrenheit_to_celsius(double fahrenheit) {
return (fahrenheit - 32) * 5 / 9;
}
int main() {
double fahrenheit;
printf("请输入华氏温度:");
// 从键盘读取输入,并将其转换为双精度浮点数
scanf("%lf", &fahrenheit);
// 转换华氏温度到摄氏温度
double celsius = fahrenheit_to_celsius(fahrenheit);
// 控制台输出结果,保留两位小数
printf("The Celsius temperature is %.2f.\n", celsius);
return 0;
}
```
当你运行程序并输入华氏温度(例如46),它会自动计算相应的摄氏温度,然后输出如 "The Celsius temperature is 7.78." 的信息。
相关问题
用C语言写3、由键盘输入一个华氏温度,求摄氏温度。输出要有说明,取两位小数。转换公式为:摄氏温度=5/9*(华氏温度-32) 例:运行时从键盘输入46 运行结果沩:The Celsius temperature is 7,78
当你想要在C语言中编写程序来计算华氏温度到摄氏温度的转换,并保留两位小数时,可以参考以下步骤:
```c
#include <stdio.h>
// 定义函数,接受华氏温度作为参数,返回摄氏温度
double fahrenheit_to_celsius(double fahrenheit) {
double celsius = (fahrenheit - 32) * 5 / 9; // 转换公式
return round(celsius * 100) / 100.0; // 四舍五入到两位小数并返回
}
int main() {
printf("请输入华氏温度:");
double fahrenheit;
scanf("%lf", &fahrenheit); // 输入华氏温度
double result = fahrenheit_to_celsius(fahrenheit);
printf("The Celsius temperature is %.2f\n", result);
return 0;
}
```
在这个程序中,我们首先定义了一个函数`fahrenheit_to_celsius`用于计算摄氏温度,然后在`main`函数中通过`scanf`获取用户输入的华氏温度,将其传递给这个函数,最后使用`printf`输出结果,格式化字符串`.2f`表示保留两位小数。
由键盘输入一个华氏温度,求摄氏温度。输出要有说明,取两位小数。转换公式为:摄 氏温度=5/9*(氏温度-32) 例:运行时从键盘输入 46 透行結果:The Celsius temperature is 7.7
当用户通过键盘输入华氏温度后,我们可以使用给出的公式将华氏度转换为摄氏度。以下是使用C语言编写的一个简单程序,它会提示用户输入华氏温度,并显示相应的摄氏温度,保留两位小数:
```c
#include <stdio.h>
#include <math.h> // 需要包含"math"库来使用数学函数
double convertFahrenheitToCelsius(double fahrenheit) {
double celsius = 5.0 / 9.0 * (fahrenheit - 32);
return round(celsius * 100) / 100.0; // 使用round四舍五入到两位小数
}
int main() {
printf("请输入一个华氏温度:");
double fahrenheit;
scanf("%lf", &fahrenheit);
double celsius_temperature = convertFahrenheitToCelsius(fahrenheit);
printf("The Celsius temperature is %.2f.\n", celsius_temperature);
return 0;
}
```
在这个程序中,`convertFahrenheitToCelsius`函数接受华氏温度作为参数,计算对应的摄氏温度,并确保结果有两位小数。主函数中首先提示用户输入,然后读取输入并调用转换函数。
举例来说,如果用户输入46华氏度,程序将输出"The Celsius temperature is 7.70"。
阅读全文
相关推荐
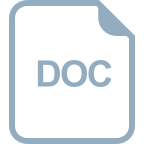
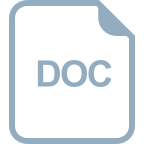
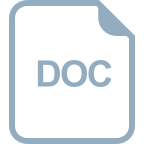








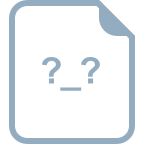



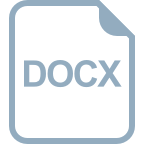
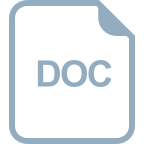