python123第六周
时间: 2024-12-27 12:27:27 浏览: 1
### Python123 Course Week 6 Materials and Content
For the sixth week of the Python123 course, students are expected to delve deeper into advanced topics that build upon foundational knowledge acquired during earlier weeks. The focus shifts towards more complex programming concepts such as object-oriented programming (OOP), file handling, and possibly an introduction to data structures like lists, dictionaries, sets, and tuples.
#### Object-Oriented Programming (OOP)
Students learn about classes and objects, encapsulation, inheritance, polymorphism, and abstraction. Understanding these principles is crucial for writing maintainable and scalable code[^2].
```python
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("Subclasses must implement abstract method")
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
dog_instance = Dog('Buddy')
print(dog_instance.speak())
```
#### File Handling
The curriculum covers reading from and writing to files using various methods available in Python. This includes opening a file, performing operations on it, and ensuring proper closure after completion.
```python
with open('example.txt', 'w') as file:
file.write('Hello World\n')
with open('example.txt', 'r') as file:
content = file.read()
print(content)
```
#### Data Structures
Advanced usage of built-in data types including list comprehensions, dictionary manipulations, set operations, and tuple unpacking techniques are explored extensively throughout this period.
```python
# List comprehension example
numbers = [i * i for i in range(5)]
print(numbers)
# Dictionary manipulation
person_info = {'name': 'Alice', 'age': 30}
person_info['city'] = 'New York'
print(person_info)
```
Before proceeding with assignments or projects related to this material, participants should review all relevant tutorials and exercises provided up until now to reinforce their understanding. Additionally, adherence to academic integrity policies remains paramount when completing coursework activities[^3].
阅读全文
相关推荐
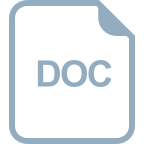
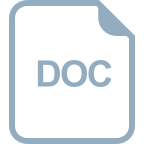
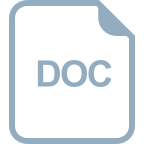



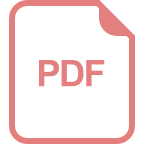
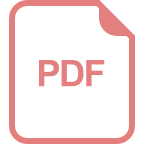
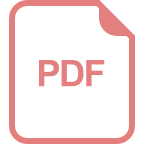
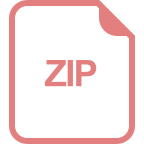
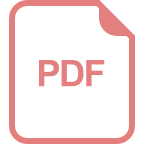
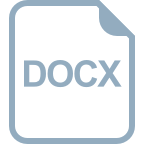
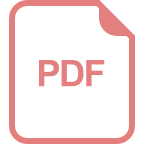
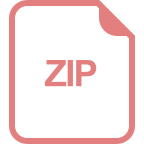




