python console subprocess.call
时间: 2023-11-22 17:35:27 浏览: 122
您好!对于在 Python 控制台中使用 `subprocess.call` 调用子进程的问题,您可以这样做:
```python
import subprocess
subprocess.call("命令", shell=True)
```
您需要将 "命令" 替换为您想要在子进程中执行的实际命令。`shell=True` 参数允许您在操作系统的命令解释器中运行命令。
请注意,`subprocess.call` 会等待子进程完成后才返回。如果您想要捕获子进程的输出或者对其进行更多的控制,您可以考虑使用 `subprocess.Popen`。
希望能帮到您!如果您还有其他问题,请随时提问。
相关问题
subprocess.call()
subprocess.call() is a method in the Python subprocess module that allows you to execute a command in a new process and waits for it to complete before returning the return code of the process.
The method takes one or more arguments, including the command to be executed and optional arguments that control how the command is executed, such as the working directory, environment variables, and input/output redirection.
Here's an example of how to use subprocess.call() to execute a command to print the output of a file:
```python
import subprocess
# Define the command to execute
cmd = ["cat", "/path/to/file.txt"]
# Execute the command and wait for it to complete
return_code = subprocess.call(cmd)
# Print the return code of the command
print(f"Command returned with code: {return_code}")
```
In this example, subprocess.call() is used to execute the "cat" command to print the contents of a file. The return code of the command is stored in the "return_code" variable and printed to the console.
subprocess.run
`subprocess.run` is a function in the Python `subprocess` module that allows you to run a command in a subprocess and capture its output. It was introduced in Python 3.5 as a simpler and more powerful replacement for the older `subprocess.call` function.
Here is an example usage of `subprocess.run`:
```python
import subprocess
result = subprocess.run(['ls', '-l'], stdout=subprocess.PIPE)
print(result.stdout.decode('utf-8'))
```
In this example, we call the `ls` command with the `-l` option to list the contents of the current directory in long format. The output of the command is captured as a byte string in the `stdout` attribute of the `CompletedProcess` object returned by `subprocess.run`. We decode the byte string to a UTF-8 string and print it to the console.
`subprocess.run` has many options that allow you to customize its behavior, such as setting the working directory, redirecting input and output, and controlling timeouts and signals. You can find more information about these options in the Python documentation.
阅读全文
相关推荐
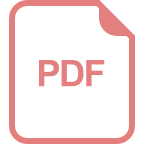
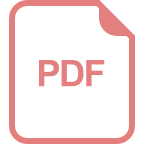
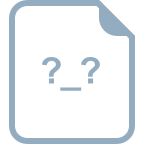
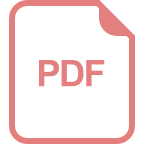
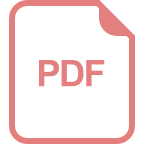
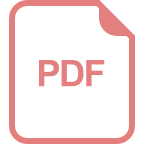
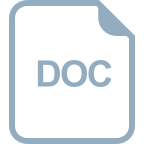
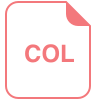
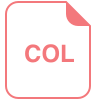
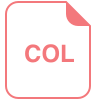
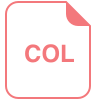
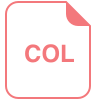
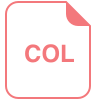
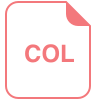

