C++如何判断文件目录是否存在
时间: 2024-12-30 10:09:26 浏览: 7
在C++中,你可以使用标准库中的`filesystem`模块(从C++17开始可用)或者`<dirent>`和`<sys/stat.h>`头文件来检查文件或目录是否存在。以下是两种常见方法:
1. 使用`std::filesystem` (推荐):
```cpp
#include <filesystem>
bool directory_exists(const std::string& path) {
try {
std::filesystem::directory_entry entry(path);
return entry.is_directory();
} catch (...) {
return false;
}
}
```
这里我们尝试打开并检查指定路径的目录入口,如果成功并且是目录,返回`true`,否则返回`false`。
2. 使用`<dirent>` 和 `<sys/stat.h>`:
```cpp
#include <dirent.h>
#include <sys/stat.h>
bool directory_exists(const std::string& path) {
struct stat buffer;
int result = stat(path.c_str(), &buffer);
if (result == 0 && S_ISDIR(buffer.st_mode)) {
return true;
} else if (errno == ENOENT) { // 指定路径不存在
return false;
} else {
perror("Failed to check directory existence");
return false;
}
}
```
在这个例子中,我们通过`stat()`系统调用来获取文件信息,如果状态位表示是目录(S_ISDIR),则返回`true`,否则处理异常或错误码。
阅读全文
相关推荐













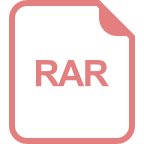

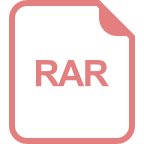