C/C++代码:斗地主
时间: 2023-11-15 07:05:57 浏览: 117
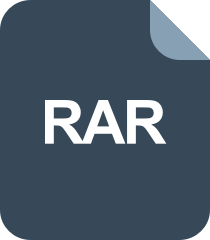
part1,斗地主源码c语言代码,c语言程序
Sure! Here's a simple implementation of a console-based "Landlord" game in C++:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
// Function to print the cards in a player's hand
void printHand(const std::vector<int>& hand) {
for (int card : hand) {
std::cout << card << " ";
}
std::cout << std::endl;
}
int main() {
// Create a deck of cards
std::vector<int> deck;
for (int i = 3; i <= 15; i++) {
deck.push_back(i);
}
// Shuffle the deck
std::random_shuffle(deck.begin(), deck.end());
// Distribute the cards to three players
std::vector<int> player1, player2, player3;
for (int i = 0; i < 51; i += 3) {
player1.push_back(deck[i]);
player2.push_back(deck[i + 1]);
player3.push_back(deck[i + 2]);
}
// Sort the cards in each player's hand
std::sort(player1.begin(), player1.end());
std::sort(player2.begin(), player2.end());
std::sort(player3.begin(), player3.end());
// Print each player's hand
std::cout << "Player 1: ";
printHand(player1);
std::cout << "Player 2: ";
printHand(player2);
std::cout << "Player 3: ";
printHand(player3);
return 0;
}
```
This code represents a simplified version of the "Landlord" game. It generates a deck of cards from 3 to 15 (where 11 represents Jack, 12 represents Queen, 13 represents King, 14 represents Ace, and 15 represents 2). The deck is then shuffled and distributed to three players. Finally, the cards in each player's hand are sorted and printed on the console.
Please note that this implementation doesn't include the game logic for playing rounds or determining the winner. It only demonstrates the initial distribution of cards.
阅读全文
相关推荐
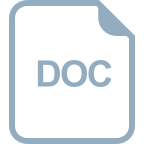
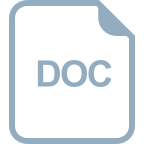
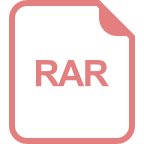
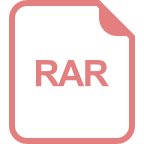
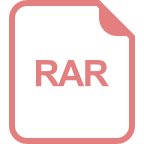
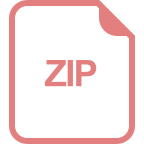
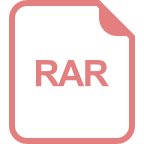
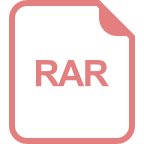
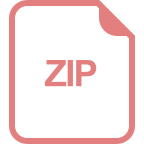
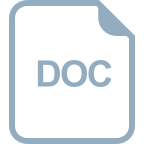
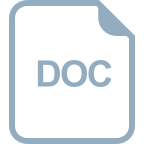



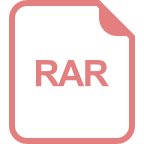
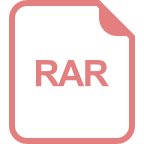
